你是java工程师,我想给emailbuild里面要发生的message是定制发送一个表格,里面行头是name和age,我应该怎么
时间: 2024-05-03 11:21:03 浏览: 18
做?
您可以使用JavaMail API来发送定制邮件带附件。首先,您需要创建一个Java类,该类将用于设置和发送电子邮件。然后,您需要创建一个表格,其中包含您要发送的信息,并保存为CSV文件。最后,您需要在Java类中编写代码来读取CSV文件中的内容,并将其添加为电子邮件的附件发送。以下是示例代码:
```
import java.util.Properties;
import javax.mail.Message;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
import javax.mail.internet.MimeBodyPart;
import javax.mail.internet.MimeMultipart;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.File;
public class EmailSender {
public static void main(String[] args) {
// Sender's email and password
String senderEmail = "your_email@example.com";
String senderPassword = "your_email_password";
// Recipient's email
String recipientEmail = "recipient_email@example.com";
// Email properties
Properties properties = new Properties();
properties.put("mail.smtp.auth", "true");
properties.put("mail.smtp.starttls.enable", "true");
properties.put("mail.smtp.host", "smtp.gmail.com");
properties.put("mail.smtp.port", "587");
// Get email session
Session session = Session.getInstance(properties, new javax.mail.Authenticator() {
protected javax.mail.PasswordAuthentication getPasswordAuthentication() {
return new javax.mail.PasswordAuthentication(senderEmail, senderPassword);
}
});
try {
// Create email message
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress(senderEmail));
message.setRecipients(Message.RecipientType.TO, InternetAddress.parse(recipientEmail));
message.setSubject("Customized email with attachment");
// Create body parts for message
MimeBodyPart messageBodyPart = new MimeBodyPart();
messageBodyPart.setText("Please see attached file for information.");
MimeBodyPart attachmentBodyPart = new MimeBodyPart();
// Read CSV file to get attachment
String csvFilePath = "/path/to/csv/file.csv";
File csvFile = new File(csvFilePath);
BufferedReader br = new BufferedReader(new FileReader(csvFile));
String line = "";
StringBuilder sb = new StringBuilder();
while ((line = br.readLine()) != null) {
sb.append(line);
sb.append(System.lineSeparator());
}
attachmentBodyPart.setContent(sb.toString(), "text/csv");
attachmentBodyPart.setFileName("data.csv");
// Add body parts to message
MimeMultipart multipart = new MimeMultipart();
multipart.addBodyPart(messageBodyPart);
multipart.addBodyPart(attachmentBodyPart);
message.setContent(multipart);
// Send message
Transport.send(message);
System.out.println("Email sent successfully.");
} catch (Exception ex) {
System.out.println("Error: " + ex.getMessage());
}
}
}
```
您需要在此代码中设置发件人和收件人的电子邮件地址以及您的发件人电子邮件密码。使用BufferedReader从CSV文件中读取信息并将其设置为电子邮件附件。在运行此代码之前,请确保您已将JavaMail API添加到您的项目中。
相关推荐
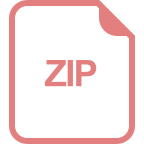
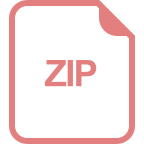
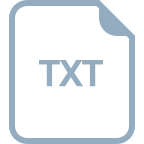














