springboot获取某个注解的bean
时间: 2024-12-04 07:27:56 浏览: 27
在Spring Boot应用中,如果你想要获取某个特定注解(比如`@Service`, `@Repository`, `@Component`, 等)修饰的Bean,你可以使用Spring的`@Autowired`、`ApplicationContext`或者`BeanDefinitionRegistry`来实现。以下是几种常见的方法:
1. **使用@Autowired注解**: 如果你需要在一个类的成员变量上自动注入符合条件的Bean,可以使用`@Autowired`注解。例如:
```java
@Service
public class MyComponent {
@Autowired
private MyAnnotatedBean myAnnotatedBean; // 自动查找带有指定注解的Bean
}
```
Spring会自动匹配并注入满足条件的Bean。
2. **手动通过ApplicationContext**: 创建`ApplicationContext`实例后,可以遍历所有可用的Bean,并检查它们的注解。例如:
```java
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public static MyAnnotatedBean getAnnotatedBean(String beanName) {
ApplicationContext context = new AnnotationConfigApplicationContext(MyConfig.class);
return context.getBean(MyAnnotatedBean.class); // 返回第一个找到的MyAnnotatedBean
}
```
这里假设`MyConfig`是一个包含其他有注解Bean的@Configuration类。
3. **使用BeanDefinitionRegistry**: 这是更底层的方法,适用于对Spring内核的直接操作。从`BeanDefinitionRegistry`中可以查询带有特定注解的所有bean定义:
```java
import org.springframework.beans.factory.config.BeanDefinition;
import org.springframework.context.support.DefaultListableBeanFactory;
// ...
DefaultListableBeanFactory factory = (DefaultListableBeanFactory) context;
List<BeanDefinition> annotatedBeans = factory.getBeanDefinitionNames()
.stream()
.filter(name -> factory.getBeanDefinition(name).isAnnotatedWith(YourAnnotation.class))
.map(factory::getBeanDefinition)
.collect(Collectors.toList());
```
这将返回一个带有关注解的Bean定义列表。
**相关问题--:**
1. Spring Boot如何处理多种类型的注解?
2. 如何避免在非Spring Bean上下文中寻找注解?
3. 在使用@Autowired注解时,如果存在多个相同注解的Bean,它会怎么选择?
阅读全文
相关推荐
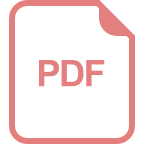
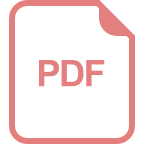
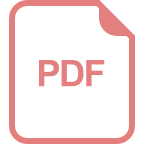















