如何编写一个程序来准确地将本地时间转换为格林尼治标准时间(GMT)并显示出来?
时间: 2024-11-05 19:34:36 浏览: 30
要编写一个程序将本地时间转换为格林尼治标准时间(GMT),你可以使用各种编程语言,这里以Python为例:
```python
from datetime import datetime, timezone
def convert_to_gmt(local_time):
# 获取当前本地时间
local_datetime = datetime.now()
# 设置格林尼治标准时间(UTC+00:00)
gmt_timezone = timezone.utc
# 转换为格林尼治时间
gmt_datetime = local_datetime.astimezone(gmt_timezone)
# 显示转换后的GMT时间
print(f"本地时间为: {local_datetime}")
print(f"格林尼治时间为: {gmt_datetime.strftime('%Y-%m-%d %H:%M:%S')}")
# 调用函数并传入本地日期和时间
convert_to_gmt()
```
在这个例子中,`datetime.now()`获取本地当前时间,然后通过`astimezone(timezone.utc)`将其转换为格林尼治时间。最后,我们使用`strftime`格式化输出时间。
相关问题
虚拟机Linux用c语言.编写时间转换程序,将当前时间转换为格林尼治时间。
在C语言中,编写一个虚拟机Linux环境下的时间转换程序需要使用`time_t`结构体、`gmtime_r`函数以及`stdio.h`库。以下是一个简单的示例,用于将当前系统时间转换为格林尼治时间:
```c
#include <stdio.h>
#include <time.h>
void convert_to_gmt() {
// 获取当前时间戳
time_t current_time = time(NULL);
// 创建一个tm结构体,用于存储转换后的日期和时间信息
struct tm *gmt_time;
char buffer[80];
// 将本地时间转换为格林尼治时间
gmtime_r(¤t_time, &gmt_time);
// 格式化输出
strftime(buffer, sizeof(buffer), "%Y-%m-%d %H:%M:%S", gmt_time);
printf("当前本地时间为: %s\n", ctime(¤t_time));
printf("格林尼治时间为: %s\n", buffer);
}
int main() {
convert_to_gmt();
return 0;
}
```
当你运行这个程序时,它会打印出当前的本地时间和对应的格林尼治时间。
如何在Delphi中通过XMLHTTP对象获取网页头信息中的时间日期,并将其转换为本地时间?
在Delphi中,你可以利用XMLHTTP对象来发送HTTP请求,并从响应的头信息中提取时间日期。这个时间通常是按照GMT(格林尼治标准时间)给出的,所以你可能需要根据本地时区进行调整以获取本地时间。以下是一个示例代码,展示如何实现这一过程:
参考资源链接:[Delphi从网页头获取网络时间日期的实现方法](https://wenku.csdn.net/doc/4tj50wj9k9?spm=1055.2569.3001.10343)
首先,需要在Delphi项目中引入`WinInet`单元,以便可以使用`TXMLHTTP`类。然后,创建一个`TXMLHTTP`对象,并调用其`Open`方法来初始化HTTP请求,设置请求方法为'GET'并指定目标URL。接下来,通过调用`Send`方法发送请求,并使用`getAllResponseHeaders`方法获取所有的响应头信息。
然后,需要在响应头信息中查找并解析Date头字段以获取时间。Date头字段通常以'GMT'结尾,格式类似于`'Sat, 26 Feb 2022 08:03:54 GMT'`。解析这个字符串后,可以使用Delphi的`DateTime`和`TTimeZone`类来转换时间到本地时区。
以下是实现的代码片段:
```delphi
uses
..., WinInet, ..., System.DateUtils, System.TimeZone;
procedure GetLocalTimeFromWebHeader(const Url: string);
var
***
***
***
***
***
***
***
***
***'GET', Url, False);
http.Send;
responseHeaders := http.GetAllResponseHeaders;
// Extract GMT date string from header
httpDateStr := ExtractDateFromHeaders(responseHeaders);
// Convert string to GMT DateTime
gmtDate := StrToDateTimeDef(httpDateStr, 0);
// Initialize TTimeZone for the GMT zone
gmtZone := TTimeZone.Create('GMT');
try
// Convert GMT DateTime to local DateTime
localDate := gmtZone.ToLocalTime(gmtDate);
finally
gmtZone.Free;
end;
// Display the local date and time
ShowMessage(FormatDateTime('yyyy/mm/dd hh:nn:ss', localDate));
finally
http.Free;
end;
end;
function ExtractDateFromHeaders(const Headers: string): string;
begin
// The implementation of this function should parse the input string
// and return the value of the 'Date' header field.
// This is a simplified example and should be replaced with
// actual string parsing logic.
Result := 'Sat, 26 Feb 2022 08:03:54 GMT';
end;
```
在这个示例中,`ExtractDateFromHeaders`函数需要你根据实际的头信息格式进行实现,用于从HTTP响应头中提取出时间字符串。`StrToDateTimeDef`函数用来将提取的字符串转换成TDateTime类型的时间。最后,使用`TTimeZone`类的`ToLocalTime`方法将GMT时间转换为本地时间。
为了更全面地掌握Delphi在HTTP网络操作方面的知识,可以参考提供的辅助资料《Delphi从网页头获取网络时间日期的实现方法》和官方文档链接,它们将帮助你深入理解并应用这些技术。
参考资源链接:[Delphi从网页头获取网络时间日期的实现方法](https://wenku.csdn.net/doc/4tj50wj9k9?spm=1055.2569.3001.10343)
阅读全文
相关推荐
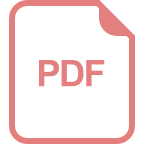
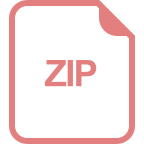
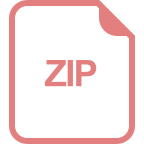













