试写一算法,删除元素非递减排列的单链表L中所有值相同的元素。即相同元素值只保留一个。c++
时间: 2024-10-07 12:08:05 浏览: 44
为了删除元素非递减排列的单链表L中所有值相同的元素,你可以采用迭代的方式遍历链表,并维护一个哈希集合(unordered_set)来跟踪已经遇到过的元素值。下面是使用C++实现的一个简单算法:
```cpp
#include <iostream>
#include <unordered_set>
// 定义单链表节点结构
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(nullptr) {}
};
void deleteDuplicates(ListNode*& head) {
if (!head) return; // 如果链表为空,直接返回
std::unordered_set<int> seen; // 存储已见值
ListNode* current = head;
while (current) {
if (seen.find(current->val) != seen.end()) { // 如果当前值已存在哈希集中
ListNode* temp = current->next;
delete current; // 删除当前节点
current = temp; // 跳过已删除的节点
} else {
seen.insert(current->val); // 添加当前值到哈希集
current = current->next;
}
}
// 更新头指针,指向最后一个非重复节点
if (head && !seen.count(head->val)) {
head = head->next;
}
}
// 打印链表辅助函数
void printList(ListNode* head) {
ListNode* temp = head;
while (temp) {
std::cout << temp->val << " -> ";
temp = temp->next;
}
std::cout << "NULL" << std::endl;
}
int main() {
ListNode* list = new ListNode(1);
list->next = new ListNode(2);
list->next->next = new ListNode(2);
list->next->next->next = new ListNode(3);
list->next->next->next->next = new ListNode(4);
list->next->next->next->next->next = new ListNode(5);
list->next->next->next->next->next = new ListNode(3);
std::cout << "Original List: ";
printList(list);
deleteDuplicates(list);
std::cout << "List after deletion of duplicates: ";
printList(list);
return 0;
}
```
这个算法首先检查每个节点的值是否已经在哈希集中,如果在则删除该节点;否则将其添加到哈希集中并移动到下一个节点。最后更新头指针,使其指向非重复部分的开始。
阅读全文
相关推荐













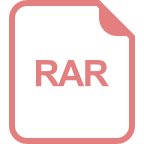


