对文档内代码增添迭代过程可视化
时间: 2024-12-23 07:17:09 浏览: 3
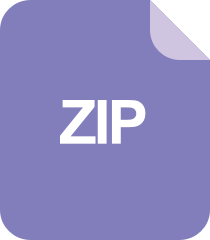
基于Python的VRED渲染自动化开发.zip

为了在遗传算法的迭代过程中实现可视化,可以使用 `matplotlib` 库来绘制每个代的最佳路径长度变化图。以下是修改后的代码:
```python
import numpy as np
import random
import matplotlib.pyplot as plt
# 距离矩阵,数据根据图片中的表格填写
distance_matrix = np.array([
[0, 11, 10, 15, 11, 9, 8, 7, 14, 11, 5, 10, 11, 16],
[11, 0, 19, 10, 11, 8, 7, 20, 14, 10, 5, 10, 16, 15],
[10, 19, 0, 5, 15, 14, 15, 7, 18, 6, 14, 17, 16, 13],
[15, 10, 5, 0, 20, 19, 14, 11, 19, 17, 6, 13, 18, 9],
[11, 11, 15, 20, 0, 5, 15, 8, 19, 14, 15, 16, 18, 19],
[9, 8, 14, 19, 5, 0, 10, 6, 16, 14, 8, 11, 14, 18],
[8, 7, 15, 14, 15, 10, 0, 5, 10, 15, 6, 5, 7, 9],
[7, 20, 7, 11, 8, 6, 5, 0, 9, 6, 8, 5, 3, 18],
[14, 14, 18, 19, 19, 16, 10, 9, 0, 17, 19, 17, 18, 7],
[11, 10, 6, 17, 14, 14, 15, 6, 17, 0, 6, 16, 15, 12],
[5, 5, 14, 6, 15, 8, 6, 8, 19, 6, 0, 10, 16, 19],
[10, 10, 17, 13, 16, 11, 5, 5, 17, 16, 10, 0, 14, 19],
[11, 16, 16, 18, 18, 14, 7, 3, 18, 15, 16, 14, 0, 19],
[16, 15, 13, 9, 19, 18, 9, 18, 7, 12, 19, 19, 19, 0]
])
# 每个客户的需求重量(单位:公斤)
demand = np.array([564, 509, 503, 350, 297, 355, 595, 446, 292, 343, 443, 233, 227])
# 车辆载重量限制(2吨 = 2000公斤)
vehicle_capacity = 2000
# 种群大小和最大代数
POP_SIZE = 100
MAX_GENERATIONS = 500
# 初始化种群,生成满足容量约束的个体
def initialize_population(pop_size, num_customers):
population = []
for _ in range(pop_size):
individual = []
remaining_customers = list(range(1, num_customers + 1)) # 客户编号1~13
while remaining_customers:
vehicle_route = [0] # 从配送中心出发 (假设0是配送中心)
load = 0
to_remove = []
for customer in remaining_customers:
if load + demand[customer - 1] <= vehicle_capacity:
vehicle_route.append(customer)
load += demand[customer - 1]
to_remove.append(customer)
for customer in to_remove:
remaining_customers.remove(customer)
vehicle_route.append(0) # 返回配送中心
individual.append(vehicle_route)
population.append(individual)
return population
# 计算每条路径的总距离,包括返回到配送中心的距离
def calculate_total_distance(individual, distance_matrix):
total_distance = 0
for route in individual:
for i in range(len(route) - 1):
total_distance += distance_matrix[route[i]][route[i+1]]
return total_distance
# 适应度函数,考虑容量约束,超载时给予惩罚
def fitness(individual, distance_matrix):
total_distance = calculate_total_distance(individual, distance_matrix)
for route in individual:
load = sum([demand[customer-1] for customer in route if customer != 0])
if load > vehicle_capacity:
total_distance *= 1.5 # 超载惩罚
return 1 / total_distance
# 选择操作,轮盘赌选择
def selection(population, fitnesses):
selected = random.choices(population, weights=fitnesses, k=len(population))
return selected
# 部分交叉操作(PMX)
def crossover(parent1, parent2):
# 简单的单点交叉操作,生成两个子代
size = min(len(parent1), len(parent2))
cx_point = random.randint(1, size - 1)
child1 = parent1[:cx_point] + parent2[cx_point:]
child2 = parent2[:cx_point] + parent1[cx_point:]
return child1, child2
# 变异操作,交换两个客户的位置
def mutate(individual):
for route in individual:
if len(route) > 2: # 确保有客户在该路径上
i, j = random.sample(range(1, len(route) - 1), 2) # 不能动配送中心
route[i], route[j] = route[j], route[i]
return individual
# 遗传算法主程序
def genetic_algorithm(pop_size=POP_SIZE, num_customers=13, generations=MAX_GENERATIONS):
population = initialize_population(pop_size, num_customers)
best_distances = []
for generation in range(generations):
# 计算适应度
fitnesses = [fitness(individual, distance_matrix) for individual in population]
# 选择操作
selected_population = selection(population, fitnesses)
# 交叉操作
next_generation = []
for i in range(0, len(selected_population), 2):
parent1 = selected_population[i]
parent2 = selected_population[i + 1] if i + 1 < len(selected_population) else selected_population[0]
child1, child2 = crossover(parent1, parent2)
next_generation.append(child1)
next_generation.append(child2)
# 变异操作
population = [mutate(individual) for individual in next_generation]
# 记录当前代最佳路径长度
best_individual = min(population, key=lambda ind: calculate_total_distance(ind, distance_matrix))
best_distance = calculate_total_distance(best_individual, distance_matrix)
best_distances.append(best_distance)
# 绘制迭代过程中的最佳路径长度变化图
plt.plot(best_distances)
plt.xlabel('Generation')
plt.ylabel('Best Distance')
plt.title('Genetic Algorithm Convergence')
plt.show()
return best_individual, best_distance
# 运行遗传算法,找到最优配送路径
best_solution, best_distance = genetic_algorithm()
print("最优配送路径:", best_solution)
print("最短总距离:", best_distance)
```
### 主要改动点:
1. **引入 `matplotlib.pyplot`**:用于绘图。
2. **记录每一代的最佳路径长度**:在每次迭代中将当前代的最佳路径长度添加到 `best_distances` 列表中。
3. **绘制图表**:在遗传算法结束时,使用 `plt.plot` 绘制每一代的最佳路径长度变化图,并显示图表。
这样,你可以直观地看到遗传算法在优化过程中如何逐渐收敛到最优解。
阅读全文
相关推荐
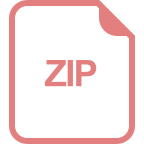
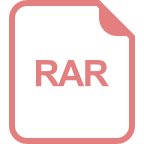
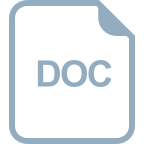
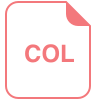
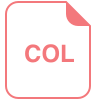
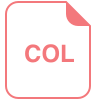
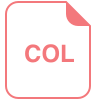
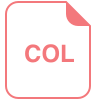
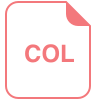
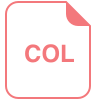
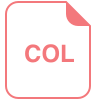
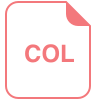
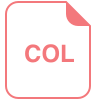
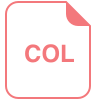
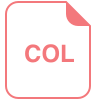
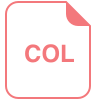
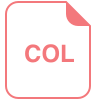
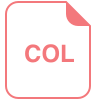