C++找到一个数组中的重复内容
时间: 2024-09-12 22:07:03 浏览: 41
在C++中找到数组中的重复内容可以通过多种方法实现,这里提供一种常见的方法,使用标准模板库(STL)中的数据结构和算法。具体步骤如下:
1. 创建一个`unordered_map`,用来存储数组中每个元素出现的次数。
2. 遍历数组,对于每个元素,增加其在`unordered_map`中的计数。
3. 再次遍历数组,检查`unordered_map`中计数大于1的元素,这些元素即为重复的内容。
示例代码如下:
```cpp
#include <iostream>
#include <vector>
#include <unordered_map>
void findDuplicates(const std::vector<int>& nums) {
std::unordered_map<int, int> countMap;
std::vector<int> duplicates;
// 计算每个元素出现的次数
for (int num : nums) {
countMap[num]++;
}
// 找出重复的元素
for (const auto& pair : countMap) {
if (pair.second > 1) {
duplicates.push_back(pair.first);
}
}
// 输出重复的元素
for (int dup : duplicates) {
std::cout << dup << " ";
}
}
int main() {
std::vector<int> nums = {1, 3, 4, 2, 2, 3, 5, 1};
findDuplicates(nums);
return 0;
}
```
阅读全文
相关推荐
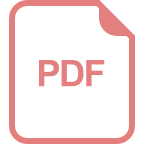
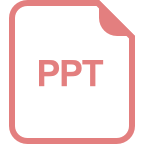
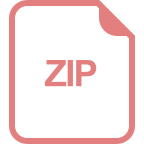















