android build.gradle.kts引用自定义gradle插件
时间: 2024-09-29 18:07:13 浏览: 9
在Android的Gradle脚本(`.kts`版本)中,引用自定义Gradle插件通常通过添加`plugins`块并指定所需的插件ID来完成。以下是基本步骤:
1. 首先,创建一个自定义的Gradle插件,它是一个`.jar`、`.aar`或者发布到Maven仓库的库。
2. 在你的项目根目录下的`build.gradle.kts`文件中,找到或添加`plugins`部分。这个部分位于`plugins { ... }`之间:
```kotlin
plugins {
id("com.example.mycustomplugin") version "1.0.0" // 自定义插件的ID和版本
}
```
在这里,`id`是你自定义插件的标识符,`version`则是对应插件的版本号。
3. 插件加载后,你可以在`plugins`块之后的`apply plugin`语句中使用自定义插件的功能:
```kotlin
plugins {
id("com.example.mycustomplugin")
}
// 使用自定义插件的某个功能
tasks.withType<MyCustomTask>() { task ->
// 设置任务属性或其他配置
}
```
4. 确保将你的自定义插件添加到项目的构建路径(`buildSrc`目录下可以存放插件源码,或者从外部引入),或者将其上传到Maven仓库以便Gradle能够查找。
相关问题
build.gradle.kts和build.gradle的区别
`build.gradle`和`build.gradle.kts`都是Gradle构建脚本的两种不同形式。`build.gradle`是使用Groovy语言编写的,而`build.gradle.kts`是使用Kotlin语言编写的。它们用于定义和配置项目构建脚本,这些脚本描述了项目构建和运行的各个阶段。
1. **语言差异**:最大的区别在于编写它们所使用的编程语言。`build.gradle`基于Groovy语言,是Gradle的默认脚本语言,拥有动态类型和灵活的语法,适合快速开发。`build.gradle.kts`则是基于Kotlin语言的脚本,Kotlin是一种静态类型、支持面向对象、函数式编程的现代语言,它提供了一种更简洁、更易于阅读和编写的语法,有助于减少配置错误。
2. **编译时间**:使用Kotlin脚本的`build.gradle.kts`在编译时通常比Groovy脚本的`build.gradle`更快。这是因为Kotlin编译器的性能优化以及其语言本身的一些特性,如明确的类型系统和更加严格的语法。
3. **集成和工具支持**:尽管`build.gradle.kts`是较新的格式,但随着Kotlin对构建和Gradle生态系统的整合,`build.gradle.kts`的支持和工具链正在不断完善。许多现代的IDE,如IntelliJ IDEA和Android Studio,都提供了良好的支持。
4. **社区和生态系统**:目前,`build.gradle`脚本因其历史悠久,在社区和生态系统方面拥有更多的资源和插件。然而,`build.gradle.kts`正逐渐获得更多的支持,许多新的Gradle插件也开始提供对它的支持。
build.gradle.kts 和build.gradle
`build.gradle.kts` 和 `build.gradle` 都是Gradle构建脚本文件,但它们分别对应的是Kotlin和Groovy这两种编程语言。Gradle是一种流行的开源构建工具,用于自动化软件项目的构建、测试和部署。
`build.gradle` 是用Groovy编写的标准版本,这是Gradle最初始的语言支持,它采用XML或Groovy语法来定义项目依赖项、构建任务等。早期的Gradle项目通常会使用`.gradle`目录下的这个文件。
而 `build.gradle.kts` 是一种更现代的选择,它是使用Kotlin编写的新式构建脚本,Kotlin是Google开发的一种静态类型的函数式编程语言,引入了更多的现代编程特性如注解和lambda表达式。由于Kotlin更接近Java的语法,因此对于熟悉Java开发者来说更容易上手,并且Kotlin编写的脚本通常更具简洁性和可读性。
两者的主要区别在于语法风格和潜在性能优化,`build.gradle.kts`有时可以提供更好的开发体验。不过,如果你的项目还没有完全转向Kotlin,或者团队成员对Groovy更为熟悉,`build.gradle`仍然是主流选择。
相关推荐
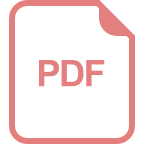
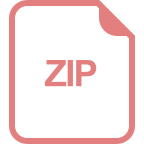
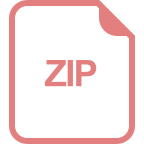












