编写一段Linux下的c语言代码,包含两个程序,要求使用命名管道来实现这两个程序间的双向通信
时间: 2024-09-13 18:09:50 浏览: 22
在Linux下使用命名管道实现两个C语言程序间的双向通信,我们需要先创建一个命名管道(也称为FIFO文件),然后在两个程序中分别以读写方式打开这个管道进行数据的发送和接收。
以下是两个简单的C语言程序示例,第一个程序(program1)写数据到管道,第二个程序(program2)从管道读数据。
首先,创建命名管道文件:
```bash
mkfifo mypipe
```
然后是第一个程序(program1.c):
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <sys/stat.h>
#include <unistd.h>
int main() {
int fd;
char *data = "Hello from program1";
char buffer[100];
// 打开FIFO文件进行写操作
fd = open("mypipe", O_WRONLY);
if (fd == -1) {
perror("open");
exit(1);
}
// 写数据到管道
if (write(fd, data, sizeof(data)) == -1) {
perror("write");
exit(1);
}
// 关闭文件描述符
close(fd);
return 0;
}
```
接下来是第二个程序(program2.c):
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <sys/stat.h>
#include <unistd.h>
int main() {
int fd;
char buffer[100];
// 打开FIFO文件进行读操作
fd = open("mypipe", O_RDONLY);
if (fd == -1) {
perror("open");
exit(1);
}
// 从管道读取数据
if (read(fd, buffer, sizeof(buffer)) == -1) {
perror("read");
exit(1);
}
printf("Received: %s\n", buffer);
// 关闭文件描述符
close(fd);
return 0;
}
```
两个程序的使用方法如下:
1. 编译这两个程序(使用gcc或其他C编译器):
```bash
gcc program1.c -o program1
gcc program2.c -o program2
```
2. 先运行program2程序,它会阻塞在读操作上,等待数据的到来。
3. 然后运行program1程序,program1会向管道写入数据。
4. program2接收到数据后,会打印出来并退出。
相关推荐
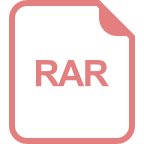
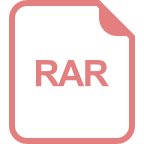
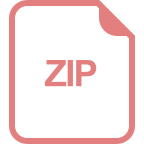














