output.append(self.loader(self.images[self.qidxs[index]])) TypeError: 'NoneType' object is not subscriptable
时间: 2024-09-24 13:11:39 浏览: 25
这个错误通常发生在尝试访问一个None类型的值时。在这个Python代码片段中,`self.images[self.qidxs[index]]`返回的是一个None对象,而`self.loader`是一个函数,试图对None对象应用`loader`方法会抛出TypeError。
`loader`函数期望一个可以被加载的图像对象,但是当`self.images[self.qidxs[index]]`的结果不是有效的图像时,它就会变成None。可能是`images`列表中的某个元素索引`index`对应的内容为空,或者`qidxs`列表中的值有问题导致了无效的索引。
解决这个问题的方法通常是检查`self.images`和`self.qidxs`是否已经初始化并且内容正确,如果可能的话,在访问之前添加条件判断:
```python
if self.images and self.qidxs:
image_to_load = self.images[self.qidxs[index]]
if image_to_load is not None:
loaded_image = self.loader(image_to_load)
else:
# 或者处理None情况,如显示错误信息或默认行为
print("Error: Image at index {} is None".format(index))
```
相关问题
这段代码为什么会报错class patient: def __init__(self, duration): self.numpatient = None self.duratin = None patient.numpatient = 30 patient.duration = duration print(patient[2].duration)报错内容为TypeError: 'type' object is not subscriptable,其中duration=[ 66 31 27 49 58 97 69 112 55 32 38 36 81 21 226 45 70 35 55 92 89 53 27 38 41 40 82 52 114 68]
在你的代码中,报错`TypeError: 'type' object is not subscriptable`是因为你尝试在类`patient`上使用索引,而类本身不支持索引操作。你应该创建一个类的实例,并在实例上使用索引。
以下是修改后的代码示例:
```python
class Patient:
def __init__(self, duration):
self.duration = duration
patients = []
duration = [66, 31, 27, 49, 58, 97, 69, 112, 55, 32, 38, 36, 81, 21, 226, 45, 70, 35, 55, 92, 89, 53, 27, 38, 41, 40, 82, 52, 114, 68]
for i in range(30):
patient = Patient(duration[i])
patients.append(patient)
print(patients[2].duration)
```
在这个修改后的代码中,我们定义了一个Patient类,并创建了一个duration列表用于初始化每个患者的持续时间。在循环中,我们创建了30个Patient实例,并将它们添加到patients列表中。然后我们打印出第三个患者(索引为2)的持续时间。
请确保在使用索引之前,你有足够的元素来访问。否则,会引发`IndexError`异常。
Python编程解释错误信息: File "E:\Python\PythonProject\日常Python学习及工具使用\知识点\类的学习\类的内部函数.py", line 14, in _listappend newlist.append[5] ~~~~~~~~~~~~~~^^^ TypeError: 'builtin_function_or_method' object is not subscriptable
这个错误信息表明在文件 "E:\Python\PythonProject\日常Python学习及工具使用\知识点\类的学习\类的内部函数.py" 的第14行发生了一个错误。具体错误是 `TypeError: 'builtin_function_or_method' object is not subscriptable`。
错误的原因是你在 `newlist.append[5]` 的代码中使用了方括号而不是括号来调用 `append` 方法。`append` 是一个方法,应该使用括号来调用,而不是方括号。所以,正确的写法应该是 `newlist.append(5)`。
以下是修正后的代码示例:
```python
class MyClass:
def __init__(self):
self.mylist = []
def _listappend(self, num):
self.mylist.append(num)
obj = MyClass()
obj._listappend(5)
print(obj.mylist) # 输出: [5]
```
在上述示例中,`_listappend` 方法将一个数值添加到 `mylist` 列表中。通过调用 `_listappend(5)`,将数值5添加到列表中。然后,通过打印 `obj.mylist` 可以验证元素5是否已经成功添加到了列表中。
阅读全文
相关推荐
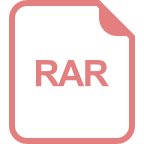
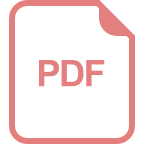
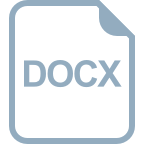
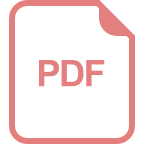
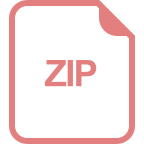
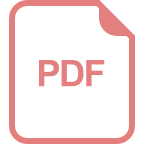
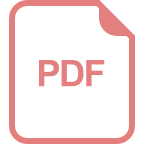
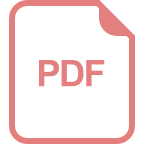
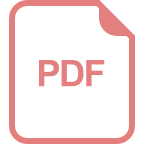
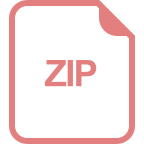
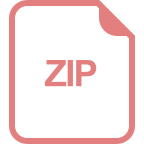
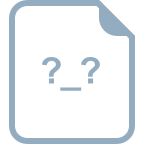
