利用java线程实现两人银行存取钱
时间: 2024-12-03 14:09:57 浏览: 5
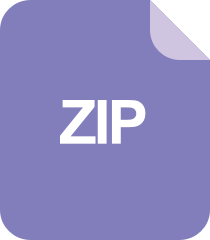
Java课程设计基于 Java 语言编写的仿 QQ 聊天工具源码+数据库

在Java中,我们可以利用线程来模拟两个人同时在银行账户上存取钱的过程,这通常涉及到并发控制和同步机制,例如`synchronized`关键字或`ReentrantLock`。下面是一个简化的示例:
```java
import java.util.concurrent.locks.Condition;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
class BankAccount {
private double balance;
private final Lock lock = new ReentrantLock();
private Condition depositCondition = lock.newCondition();
private Condition withdrawCondition = lock.newCondition();
public void deposit(double amount) {
lock.lock();
try {
if (amount > 0) {
balance += amount;
System.out.println("Thread " + Thread.currentThread().getName() + " deposited: " + amount);
depositCondition.signalAll(); // 其他尝试存款的线程可以继续
}
} finally {
lock.unlock();
}
}
public synchronized void withdraw(double amount) {
while (balance < amount) {
try {
withdrawCondition.await(); // 等待直到有足够的余额
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
if (amount <= balance) {
balance -= amount;
System.out.println("Thread " + Thread.currentThread().getName() + " withdrew: " + amount);
} else {
System.out.println("Insufficient balance.");
}
}
}
public class BankTransaction {
public static void main(String[] args) throws InterruptedException {
BankAccount account = new BankAccount();
Thread t1 = new Thread(() -> account.deposit(500));
Thread t2 = new Thread(() -> account.withdraw(200));
t1.start();
t2.start();
t1.join();
t2.join();
}
}
```
在这个例子中,我们创建了一个`BankAccount`类,它有共享的`balance`变量和两个条件变量`depositCondition`和`withdrawCondition`,用于线程间的协作。当存款线程完成操作后会唤醒等待的取款线程,而取款线程只有在账户余额足够的情况下才会允许执行。
阅读全文
相关推荐
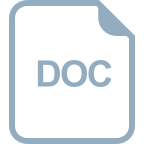
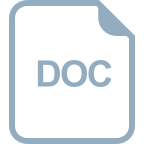
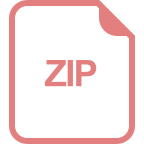
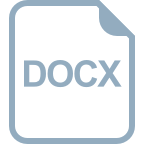
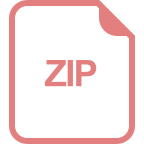
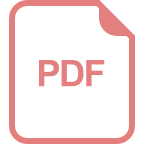
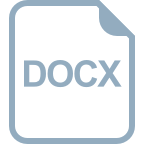
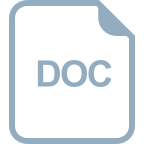
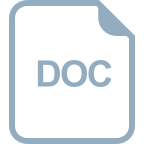
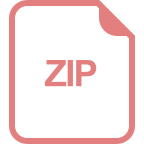
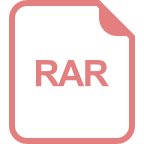
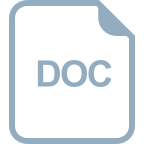
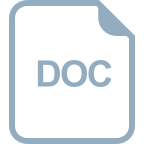
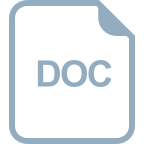
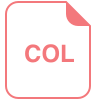
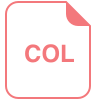
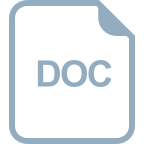
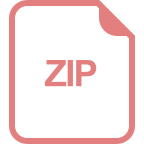