1.创建了一个学生成绩管理系统,能够添加学生学号及其成绩,并将数据保存到文本文件中,可以查询学号对应的成绩,另外可以统计成绩的平分,最高分和最低分。(python代码)
时间: 2024-11-15 16:23:22 浏览: 13
在Python中,你可以使用内置的数据结构如字典存储学生的成绩信息,并结合文件操作来实现这个学生成绩管理系统。以下是一个简化的代码示例:
```python
# 定义一个函数来读取或创建成绩数据
def read_or_create_scores(filename):
try:
with open(filename, 'r') as file:
return {int(line.split()[0]): float(line.split()[1]) for line in file}
except FileNotFoundError:
return {}
# 添加新分数
def add_score(scores, student_id, score):
scores[student_id] = score
save_scores(scores, filename)
# 保存所有分数到文件
def save_scores(scores, filename):
with open(filename, 'w') as file:
for student_id, score in sorted(scores.items()):
file.write(f"{student_id} {score}\n")
# 查询成绩
def get_score(scores, student_id):
if student_id in scores:
return scores[student_id]
else:
print(f"Student ID {student_id} not found.")
# 统计平均分、最高分和最低分
def stats(scores):
total = sum(scores.values())
average = total / len(scores) if scores else None
max_score = max(scores.values()) if scores else None
min_score = min(scores.values()) if scores else None
return average, max_score, min_score
# 示例使用
scores = read_or_create_scores("scores.txt")
add_score(scores, 12345, 85.0)
print(get_score(scores, 12345))
average, highest, lowest = stats(scores)
print(f"Average: {average}, Highest: {highest}, Lowest: {lowest}")
```
在这个例子中,我们假设`scores.txt`文件的格式是每行包含一个学号和对应的分数,如果没有文件存在,它会自动创建。每次添加新成绩后,都会立即保存到文件。
阅读全文
相关推荐












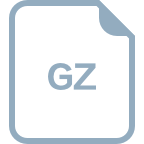

