编写一个shell程序,将指定目录及其子目录中的包含字符串root的文本文件找出来
时间: 2024-09-27 09:10:10 浏览: 45
在Shell脚本中编写查找含有特定字符串(如"root")的文本文件的程序,可以使用`find`命令配合通配符`*`。下面是一个简单的例子:
```bash
#!/bin/bash
# 指定要搜索的根目录
search_dir="/path/to/your/directory"
# 使用find命令查找包含字符串"root"的文本文件
grep_root_files=$(find $search_dir -type f -name "*root*" -exec grep -l "root" {} \;)
# 输出找到的文件路径
echo "Found the following files containing 'root':"
echo "$grep_root_files"
```
在这个脚本中,`find`命令会递归地搜索指定目录及其所有子目录(`-type f`表示只查找普通文件),然后通过`-name "*root*"`匹配包含"root"的文件名。`-exec grep -l "root" {} \;`用于在找到的每个文件中查找"root"并返回包含该字符串的文件的完整路径。
相关问题
User 利用POSIX API(文件操作也可以使用ANSI C标准I/O库)编程实现cp –r命令,支持将源路径(目录)中的所有文件和子目录,以及子目录中的所有内容,全部拷贝到目标路径(目录)中。UNIX/Linux中在shell中输入命令名(可执行文件名)来启动程序,在命令名(可执行文件名)之后可以跟随一系列字符串(通过空格分割),这些字符串就是命令行参数 格式为:cp [参数] <源文件路径> <目标文件路径> cp /usr/local/src/main.c /root/main.c(文件到文件复制) cp /usr/local/src/main.c /root (文件到目录复制) cp –r /usr/local/src /root(递归复制,用于目录到目录的复制)
ChitGPT:
您好!关于您的问题,以下是实现cp –r命令的C代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <dirent.h>
void copy_file(char *src, char *dest) {
int src_fd, dest_fd;
ssize_t num_read;
char buf[BUFSIZ];
src_fd = open(src, O_RDONLY);
if (src_fd == -1) {
fprintf(stderr, "Failed to open %s\n", src);
exit(1);
}
dest_fd = creat(dest, S_IRUSR | S_IWUSR | S_IRGRP | S_IROTH);
if (dest_fd == -1) {
fprintf(stderr, "Failed to create %s\n", dest);
exit(1);
}
while ((num_read = read(src_fd, buf, BUFSIZ)) > 0) {
if (write(dest_fd, buf, num_read) != num_read) {
fprintf(stderr, "Failed to write to %s\n", dest);
exit(1);
}
}
if (num_read == -1) {
fprintf(stderr, "Failed to read from %s\n", src);
exit(1);
}
if (close(src_fd) == -1) {
fprintf(stderr, "Failed to close %s\n", src);
exit(1);
}
if (close(dest_fd) == -1) {
fprintf(stderr, "Failed to close %s\n", dest);
exit(1);
}
}
void copy_dir(char *src, char *dest) {
DIR *dp;
struct dirent *entry;
struct stat statbuf;
char src_path[PATH_MAX], dest_path[PATH_MAX];
dp = opendir(src);
if (dp == NULL) {
fprintf(stderr, "Failed to open directory %s\n", src);
exit(1);
}
if (mkdir(dest, S_IRWXU | S_IRWXG | S_IROTH | S_IXOTH) == -1) {
fprintf(stderr, "Failed to create directory %s\n", dest);
exit(1);
}
while ((entry = readdir(dp)) != NULL) {
if (strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0) {
continue;
}
snprintf(src_path, PATH_MAX, "%s/%s", src, entry->d_name);
snprintf(dest_path, PATH_MAX, "%s/%s", dest, entry->d_name);
if (lstat(src_path, &statbuf) == -1) {
fprintf(stderr, "Failed to stat %s\n", src_path);
exit(1);
}
if (S_ISREG(statbuf.st_mode)) {
copy_file(src_path, dest_path);
} else if (S_ISDIR(statbuf.st_mode)) {
copy_dir(src_path, dest_path);
}
}
if (closedir(dp) == -1) {
fprintf(stderr, "Failed to close directory %s\n", src);
exit(1);
}
}
int main(int argc, char *argv[]) {
char *src_path, *dest_path;
if (argc != 3) {
fprintf(stderr, "Usage: %s <source> <destination>\n", argv[0]);
exit(1);
}
src_path = argv[1];
dest_path = argv[2];
struct stat statbuf;
if (lstat(src_path, &statbuf) == -1) {
fprintf(stderr, "Failed to stat %s\n", src_path);
exit(1);
}
if (S_ISREG(statbuf.st_mode)) {
copy_file(src_path, dest_path);
} else if (S_ISDIR(statbuf.st_mode)) {
copy_dir(src_path, dest_path);
}
return 0;
}
```
该代码实现了cp –r命令的递归复制功能,可以将源路径(目录)中的所有文件和子目录,以及子目录中的所有内容,全部拷贝到目标路径(目录)中。在命令行中,用户可以输入如下命令来执行该程序:
```
./cp –r /path/to/source /path/to/destination
```
其中,/path/to/source是源路径(目录),/path/to/destination是目标路径(目录)。
在目录a中查找所有文件,哪些出现字符串RK_FUNC_GPIO
在目录`a`中查找所有包含字符串`RK_FUNC_GPIO`的文件,通常需要通过操作系统提供的命令行工具或者编程语言库来实现。这里提供几个常见的操作方法:
1. **Linux / Unix Shell**:
使用`grep`命令可以在目录下递归搜索文件内容:
```
grep -r "RK_FUNC_GPIO" /path/to/directory/a
```
这里 `/path/to/directory/a` 需替换为实际的目录路径。
2. **Windows Command Prompt**:
可以使用 `dir /s /b * RK_FUNC_GPIO` (加上 `/S` 表示搜索子目录) 后跟回车,然后筛选结果。
3. **Python**:
如果你在Python环境下,可以使用`os`和`re`模块:
```python
import os
import re
def find_files_with_string(directory, search_string):
for root, dirs, files in os.walk(directory):
for file in files:
if re.search(r"RK_FUNC_GPIO", open(os.path.join(root, file), 'r').read()):
print(os.path.join(root, file))
find_files_with_string("/path/to/directory/a", "RK_FUNC_GPIO")
```
4. **PowerShell**:
类似地,你可以使用`Get-ChildItem`和`Select-String`:
```
Get-ChildItem -Path C:\path\to\directory\a -Recurse | Where-Object { $_.Content -match "RK_FUNC_GPIO" }
```
请注意,以上操作可能会列出大量文件,建议结合具体需求进一步筛选结果。同时,你需要确保有权限访问指定的目录。如果你想要查看匹配文件的详细信息,而不只是文件名,可以根据相应命令进行调整。
阅读全文
相关推荐
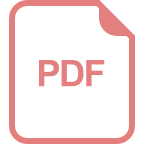
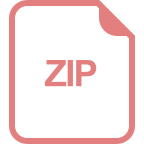
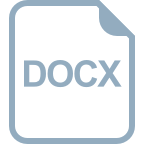
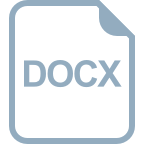
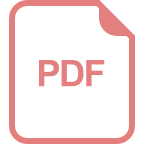
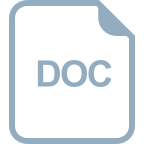
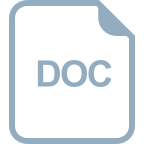
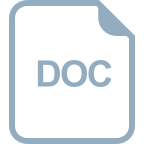
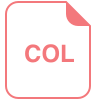
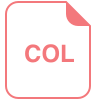
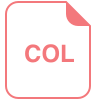
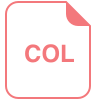
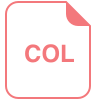
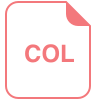
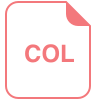
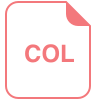