python多线程打地鼠 树莓派
时间: 2023-10-18 17:06:26 浏览: 42
好的,针对您的问题,我可以给出以下思路:
1. 首先需要安装 GPIO 库,用于控制树莓派的 GPIO 引脚。
2. 然后可以使用 Python 的 threading 模块创建多个线程,每个线程控制一个地鼠。
3. 在每个线程中,可以使用 GPIO 库控制对应的引脚,使地鼠出现或消失。
4. 可以使用 time 模块控制地鼠出现和消失的时间间隔。
下面是一个简单的示例代码:
```python
import RPi.GPIO as GPIO
import threading
import time
# 地鼠引脚列表
mole_pins = [11, 12, 13, 15, 16, 18]
# 初始化 GPIO
GPIO.setmode(GPIO.BOARD)
for pin in mole_pins:
GPIO.setup(pin, GPIO.OUT)
# 地鼠线程类
class MoleThread(threading.Thread):
def __init__(self, pin):
super().__init__()
self.pin = pin
self.stop_event = threading.Event()
def run(self):
while not self.stop_event.is_set():
# 地鼠出现
GPIO.output(self.pin, GPIO.HIGH)
time.sleep(0.5)
# 地鼠消失
GPIO.output(self.pin, GPIO.LOW)
time.sleep(0.5)
def stop(self):
self.stop_event.set()
# 创建地鼠线程
mole_threads = []
for pin in mole_pins:
mole_thread = MoleThread(pin)
mole_threads.append(mole_thread)
# 启动地鼠线程
for mole_thread in mole_threads:
mole_thread.start()
# 等待一段时间后停止地鼠线程
time.sleep(10)
for mole_thread in mole_threads:
mole_thread.stop()
# 清理 GPIO
GPIO.cleanup()
```
相关推荐
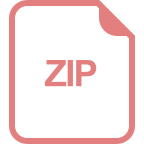














