VS Studio C# 调用C++Builder 生成的dll 的方法
时间: 2024-02-23 16:02:54 浏览: 105
在 VS Studio 中调用 C++Builder 生成的 DLL,可以按照以下步骤进行操作:
1. 在 C++Builder 中,创建一个动态链接库项目,并实现需要调用的函数。
2. 在 C++Builder 中,编译生成 DLL 文件,并记住 DLL 文件的路径。
3. 在 VS Studio 中,创建一个 C# 项目,并在项目中添加对 DLL 文件的引用。
4. 在 C# 代码中,使用 `DllImport` 特性声明需要调用的函数。
例如,如果 C++Builder 中的 DLL 文件中包含以下函数:
```c++
extern "C" __declspec(dllexport) int __stdcall Add(int a, int b);
```
则在 C# 代码中,可以声明该函数如下:
```c#
[DllImport("path/to/dll/file.dll", CallingConvention = CallingConvention.StdCall)]
public static extern int Add(int a, int b);
```
其中 `path/to/dll/file.dll` 是 DLL 文件的路径,`CallingConvention.StdCall` 指定了调用约定为 stdcall。
5. 在 C# 代码中,可以调用该函数并获取返回值,例如:
```c#
int result = Add(1, 2);
```
这样就可以在 C# 项目中成功调用 C++Builder 生成的 DLL 了。
相关问题
VS STUDIO C# 调用 C++Builder生成的Dll
在VS Studio C#中调用C++Builder生成的DLL,同样可以使用P/Invoke技术。
首先,在C++Builder中定义一个导出函数,例如:
```cpp
extern "C" __declspec(dllexport) int Add(int a, int b)
{
return a + b;
}
```
然后,将该函数编译成DLL库文件(例如MyCppLibrary.dll)。确保在编译时使用stdcall调用约定,以便能够与C#程序进行正确的交互。
接下来,在C#中使用DllImport特性声明该函数,并调用它:
```csharp
using System;
using System.Runtime.InteropServices;
class Program
{
[DllImport("MyCppLibrary.dll")]
public static extern int Add(int a, int b);
static void Main(string[] args)
{
int result = Add(1, 2);
Console.WriteLine(result); // 输出 "3"
}
}
```
在上面的示例中,DllImport特性指定了C++Builder生成的DLL库文件名和函数名。调用Add函数时,C#会自动将参数和返回值转换为正确的类型。
需要注意的是,C++Builder和C#程序都需要使用相同的ABI(Application Binary Interface)。如果C++Builder使用了其他调用约定,需要在DllImport特性中指定 CallingConvention 参数。
阅读全文
相关推荐





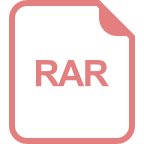
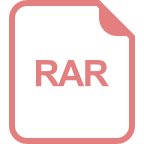
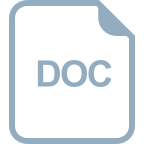

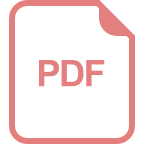






