vector中count函数的用法
时间: 2023-09-20 17:07:30 浏览: 208
`std::count` 是 C++ STL 中的一个算法函数,它的作用是计算指定区间内等于某个给定值的元素个数。
函数定义如下:
```c++
template< class InputIt, class T >
typename iterator_traits<InputIt>::difference_type
count( InputIt first, InputIt last, const T& value );
```
其中,`first` 和 `last` 分别表示指向区间的起始位置和末尾位置的迭代器,`value` 表示要计数的元素值。
函数返回值是指定区间内等于 `value` 的元素个数。
以下是一个示例:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec{1, 2, 3, 2, 1, 4, 2};
int count = std::count(vec.begin(), vec.end(), 2); // 统计 2 的个数
std::cout << "count = " << count << std::endl; // 输出 3
return 0;
}
```
在上述代码中,`count` 函数统计了 `vec` 向量中等于 2 的元素个数,结果为 3。
相关问题
c语言中count函数的用法
c语言中没有内置的count函数,可能是指STL中的count函数,或者是自定义的函数。
1. STL中的count函数:
count函数是C++标准库中的一个函数,可以用来统计元素在容器中出现的次数。其用法为:
```
#include <algorithm> //头文件
std::count(容器的起始地址, 容器的结束地址, 待统计的元素);
```
例如:
```
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
int main()
{
vector<int> v = {1, 2, 3, 2, 4, 2, 5};
int count = std::count(v.begin(), v.end(), 2);
cout << "2出现的次数为:" << count << endl; //输出:2出现的次数为:3
return 0;
}
```
2. 自定义的函数:
也可以自定义函数实现统计元素在数组中出现的次数。例如:
```
#include <stdio.h>
int count(int arr[], int len, int target)
{
int cnt = 0;
for (int i = 0; i < len; i++)
{
if (arr[i] == target)
cnt++;
}
return cnt;
}
int main()
{
int arr[] = {1, 2, 3, 2, 4, 2, 5};
int len = sizeof(arr) / sizeof(int);
int target = 2;
int cnt = count(arr, len, target);
printf("%d出现的次数为:%d\n", target, cnt); //输出:2出现的次数为:3
return 0;
}
```
vector count
在C++中,可以使用count函数来计算一个vector中特定元素的个数。这个函数的使用方式是count(vector.begin(), vector.end(), target),其中vector是要进行计数的vector容器,target是要计数的目标元素。\[1\]另外,也可以使用std::count函数来实现相同的功能,使用方式是std::count(vector.begin(), vector.end(), target)。\[2\]这两种方法都会返回目标元素在vector中出现的次数。
另外,如果你想在构造函数中向vector中添加数据,可以使用push_back函数将数据添加到vector中。\[3\]这样,你就可以在构造函数中输入数据并将其存储在vector中了。
#### 引用[.reference_title]
- *1* [【C++STL】vector的count()和count_if()函数的用法](https://blog.csdn.net/m0_38033475/article/details/79561369)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^koosearch_v1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* [C++ std::vector count统计某个元素个数 是否存在某个值](https://blog.csdn.net/u013288190/article/details/120686563)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^koosearch_v1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [向量数组vector的构造函数写法以及count函数的使用](https://blog.csdn.net/pxl20171799/article/details/81227716)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^koosearch_v1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
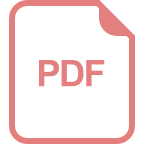
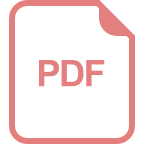
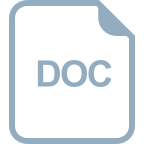












