H265编码过程与解码过程,请用C语言举例并解释
时间: 2023-06-19 10:08:12 浏览: 599
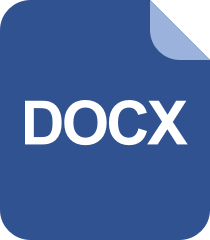
从码流获取H264、H265的分辨率信息

H.265 (High Efficiency Video Coding, HEVC) 是一种现代视频编码标准,它采用先进的压缩算法,能够以更低的比特率提供更高质量的视频。下面是 H.265 编码和解码的基本过程,以及使用 C 语言的示例代码。
## 编码过程
1. 分解图像:将输入视频分解成一系列帧,每帧由若干个宏块 (CU) 组成。
```c
// 读取视频帧
AVFrame *frame = av_frame_alloc();
if (!frame) {
return -1;
}
int ret = avcodec_receive_frame(codec_context, frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
return ret;
} else if (ret < 0) {
return -1;
}
// 分解帧为宏块
for (int y = 0; y < frame->height; y += CU_SIZE) {
for (int x = 0; x < frame->width; x += CU_SIZE) {
uint8_t *data = frame->data[0] + y * frame->linesize[0] + x;
encode_cu(data, frame->linesize[0], CU_SIZE);
}
}
```
2. 预测和变换:对每个宏块进行预测和变换操作,以减少空间冗余性和时间冗余性。
```c
void encode_cu(uint8_t *data, int stride, int size) {
uint8_t *src = data;
uint8_t *dst = malloc(size * size);
// 预测
predict(src, stride, dst, size);
// 变换
transform(dst, size);
// 编码
encode(dst, size);
free(dst);
}
```
3. 量化和编码:将变换系数量化为整数,然后使用熵编码算法将整数编码为比特流。
```c
void encode(uint8_t *data, int size) {
int coeffs[size * size];
quantize(data, size, coeffs);
entropy_encode(coeffs, size);
}
```
## 解码过程
1. 解码比特流:将压缩的比特流解码为整数系数。
```c
void decode(uint8_t *data, int size) {
int coeffs[size * size];
entropy_decode(coeffs, size);
dequantize(coeffs, size, data);
}
```
2. 反变换和反预测:将整数系数反量化为浮点数,然后进行反变换和反预测操作,以重构原始图像。
```c
void decode_cu(uint8_t *data, int stride, int size) {
uint8_t *dst = data;
uint8_t *src = malloc(size * size);
// 解码
decode(src, size);
// 反变换
inverse_transform(src, size);
// 反预测
inverse_predict(src, size, dst, stride);
free(src);
}
```
3. 组合图像:将所有宏块组合成完整的视频帧。
```c
// 组合帧
AVFrame *frame = av_frame_alloc();
if (!frame) {
return -1;
}
for (int y = 0; y < frame->height; y += CU_SIZE) {
for (int x = 0; x < frame->width; x += CU_SIZE) {
uint8_t *data = frame->data[0] + y * frame->linesize[0] + x;
decode_cu(data, frame->linesize[0], CU_SIZE);
}
}
```
阅读全文
相关推荐
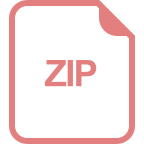
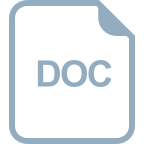
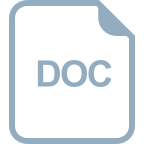


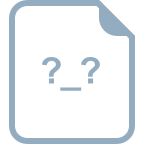
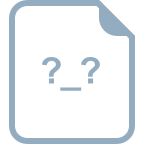
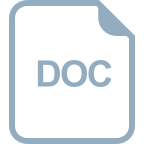
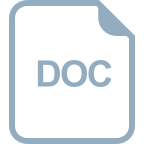
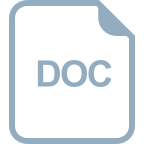
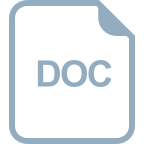
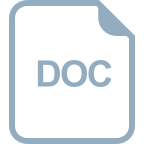
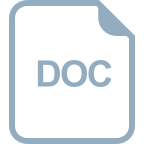
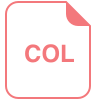
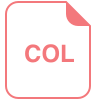