使用二进制遗传算法求函数f(x)=∑_(i=1)^30▒x^2 的最大值。其中,x 为二进制设计变量,即x={0, 1} ,并写出matlab中的代码,交叉算子用两点交叉来做,选择算子用两两竞争法来做。
时间: 2024-12-13 17:23:57 浏览: 14
二进制遗传算法是一种用于解决优化问题的搜索方法,尤其适用于离散优化问题。在您的例子中,我们想要找到函数 f(x) = ∑(x_i^2),其中 x 是由 30 个二进制位组成的设计变量(x_i ∈ {0, 1})。为了找到这个函数的最大值,我们可以使用以下步骤创建 MATLAB 代码:
1. 初始化种群(Population):随机生成一些二进制编码的个体作为初始种群。
2. 计算适应度:对于每个个体,计算其对应函数值 f(x),适应度通常是该函数值的倒数,以便较小的 f(x) 表示更好的解。
3. 选择操作:采用两两竞争法(Tournament Selection),从种群中选取部分个体参与下一轮竞争。
4. 交叉操作:使用两点交叉(Two-point Crossover)对选定的个体进行交叉,生成新的候选解。
5. 变异操作:随机地改变新产生的部分位,保持二进制属性。
6. 更新种群:替换适应度较差的部分个体,将新的候选解加入到种群中。
7. 重复步骤3-6直到达到预设的迭代次数或找到满足条件的解。
下面是一个简化的 MATLAB 代码示例:
```Matlab
function [bestFitness, bestSolution] = binaryGA(fitnessFunction, nVariables, popSize, maxGen, crossoverProb)
% 初始化种群
population = randi([0 1], popSize, nVariables);
% 初始化最佳解
bestFitness = Inf;
bestSolution = [];
% 迭代
for gen = 1:maxGen
% 计算适应度
fitness = zeros(size(population));
for i = 1:popSize
fitness(i) = 1 / fitnessFunction(population(i, :));
end
% 选择操作
selectedParents = tournamentSelection(fitness, popSize);
% 交叉操作
children = twoPointCrossover(selectedParents, crossoverProb);
% 变异操作
mutatedChildren = bitFlipMutation(children);
% 更新种群
newPopulation = [population(selectedParents, :); mutatedChildren];
% 更新最佳解
if min(fitness(newPopulation)) < bestFitness
bestFitness = min(fitness(newPopulation));
bestSolution = newPopulation(min(find(fitness == bestFitness)), :);
end
% 更新种群
population = newPopulation;
end
end
% 两点交叉函数
function children = twoPointCrossover(parents, crossoverProb)
nParents = size(parents, 1);
crossoverMask = randsample(nParents, round(nParents * crossoverProb), 'replace');
children = [];
for i = 1:nParents
if ismember(i, crossoverMask)
% 交换两个点后的子串
crossPoint1 = randsample(1:end-i+1, 1);
crossPoint2 = crossPoint1 + randsample(end-crossPoint1+1, 1);
child = [parents(i, 1:crossPoint1) parents(i, crossPoint2:end)];
else
child = parents(i, :);
end
children = [children; child];
end
end
% 比赛选择函数
function selectedParents = tournamentSelection(fitness, nParents)
selectedParents = zeros(1, nParents);
for i = 1:nParents
competitors = randsample(1:length(fitness), 2);
if fitness(competitors(1)) < fitness(competitors(2))
selectedParents(i) = competitors(1);
else
selectedParents(i) = competitors(2);
end
end
end
% 随机变异函数
function mutatedChildren = bitFlipMutation(children, mutationProb)
nChildren = size(children, 1);
mutationMask = randsample(nChildren, round(nChildren * mutationProb), 'replace');
mutatedChildren = children;
for i = 1:nChildren
if ismember(i, mutationMask)
mutatedChildren(i, randsample(1:size(mutatedChildren(i,:), 2))) = 1 - mutatedChildren(i, randsome(1:size(mutatedChildren(i,:), 2)));
end
end
end
% 定义目标函数
fitnessFunction = @(x) sum(x .^ 2);
% 调用遗传算法
[~, bestSolution] = binaryGA(fitnessFunction, 30, 100, 1000, 0.8); % 设置参数如需调整
% 打印结果
fprintf('Best solution found: %s\n', mat2str(bestSolution));
fprintf('Maximum value of the function: %.2f\n', fitnessFunction(bestSolution));
```
请注意,上述代码只是一个基础版本,实际应用可能需要添加更多细节,例如边界检查、早停等条件。运行此代码前,请确保已经安装了 MATLAB 并有必要的工具箱。此外,因为是文本环境,无法直接运行代码,你需要在 MATLAB 环境中编写和执行。
阅读全文
相关推荐










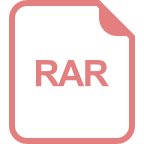




