using System.Threading; Thread thread1 = new Thread(new ThreadStart(setDatagridview11)); Thread thread2 = new Thread(new ThreadStart(setDatagridview22)); thread1.Start(); thread2.Start(); thread1.Join(); thread2.Join(); 方法有参数 怎么写
时间: 2023-08-14 17:06:32 浏览: 47
如果 `setDatagridview11` 和 `setDatagridview22` 方法需要参数,可以使用 `ParameterizedThreadStart` 委托来启动线程,并将参数传递给方法。
例如,如果 `setDatagridview11` 方法需要一个名为 `data` 的字符串参数,可以这样启动线程:
```
string data = "这是传递给 setDatagridview11 方法的参数";
Thread thread1 = new Thread(new ParameterizedThreadStart(setDatagridview11));
thread1.Start(data);
```
在 `setDatagridview11` 方法中,需要将参数转换为正确的类型:
```
private void setDatagridview11(object data)
{
string dataString = (string)data;
// 使用传递进来的参数
}
```
同样的,对于 `setDatagridview22` 方法也可以这样处理。
相关问题
在thread1和thread2中创建多个线程 参考using System.Threading; Thread thread1 = new Thread(new ThreadStart(setDatagridview11)); Thread thread2 = new Thread(new ThreadStart(setDatagridview22)); thread1.Start(); thread2.Start(); thread1.Join(); thread2.Join();
如果需要在 thread1 和 thread2 中创建多个线程,可以在 setDatagridview11 和 setDatagridview22 方法中使用 Thread 类来创建新线程。然后使用 Start 方法来启动新线程,并使用 Join 方法等待它们完成。
下面是一个示例代码:
```
using System;
using System.Threading;
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Main thread started.");
Thread thread1 = new Thread(new ThreadStart(setDatagridview11));
Thread thread2 = new Thread(new ThreadStart(setDatagridview22));
thread1.Start();
thread2.Start();
thread1.Join();
thread2.Join();
Console.WriteLine("Main thread finished.");
Console.ReadKey();
}
static void setDatagridview11()
{
Console.WriteLine("setDatagridview11 started.");
for (int i = 0; i < 5; i++)
{
Thread childThread = new Thread(new ParameterizedThreadStart(ChildThreadFunc));
childThread.Start(i);
}
Console.WriteLine("setDatagridview11 finished.");
}
static void setDatagridview22()
{
Console.WriteLine("setDatagridview22 started.");
for (int i = 0; i < 5; i++)
{
Thread childThread = new Thread(new ParameterizedThreadStart(ChildThreadFunc));
childThread.Start(i + 5);
}
Console.WriteLine("setDatagridview22 finished.");
}
static void ChildThreadFunc(object index)
{
Console.WriteLine("Child thread {0} started.", index);
Thread.Sleep(1000);
Console.WriteLine("Child thread {0} finished.", index);
}
}
```
在这个示例中,setDatagridview11 和 setDatagridview22 方法分别创建了 5 个新线程,并在其中调用了 ChildThreadFunc 方法。在 Main 方法中,启动 thread1 和 thread2 线程,并使用 Join 方法等待它们完成。运行程序后,你将会看到如下输出:
```
Main thread started.
setDatagridview11 started.
setDatagridview22 started.
Child thread 0 started.
Child thread 5 started.
Child thread 1 started.
Child thread 6 started.
Child thread 2 started.
Child thread 7 started.
Child thread 3 started.
Child thread 8 started.
Child thread 4 started.
Child thread 9 started.
Child thread 0 finished.
Child thread 5 finished.
Child thread 1 finished.
Child thread 6 finished.
Child thread 2 finished.
Child thread 7 finished.
Child thread 3 finished.
Child thread 8 finished.
Child thread 4 finished.
Child thread 9 finished.
setDatagridview11 finished.
setDatagridview22 finished.
Main thread finished.
```
c# 可以动态创建childThread嘛?并且join 参考using System.Threading; Thread thread1 = new Thread(new ThreadStart(setDatagridview11)); Thread thread2 = new Thread(new ThreadStart(setDatagridview22)); thread1.Start(); thread2.Start(); thread1.Join(); thread2.Join();
是的,C#也可以使用类似的方式来动态创建子线程并等待其结束。以下是一个类似的C#示例代码:
```csharp
using System;
using System.Threading;
class Program
{
static void Main(string[] args)
{
Thread thread1 = new Thread(new ThreadStart(setDatagridview11));
Thread thread2 = new Thread(new ThreadStart(setDatagridview22));
thread1.Start();
thread2.Start();
// 等待所有子线程结束
thread1.Join();
thread2.Join();
Console.WriteLine("All threads finished");
}
static void setDatagridview11()
{
Console.WriteLine("Thread 1 started");
// do some work
Console.WriteLine("Thread 1 finished");
}
static void setDatagridview22()
{
Console.WriteLine("Thread 2 started");
// do some work
Console.WriteLine("Thread 2 finished");
}
}
```
这段代码会创建两个子线程,分别执行setDatagridview11()和setDatagridview22()方法。主线程会等待所有子线程执行完毕后才会输出"All threads finished"。
相关推荐
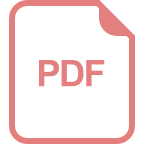
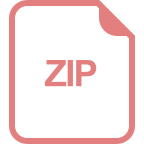
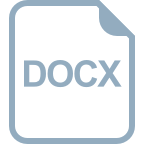













