C#读取onnx节点数据
时间: 2023-08-24 20:05:03 浏览: 189
要在C#中读取ONNX节点数据,可以使用Microsoft.ML.OnnxRuntime库。以下是一个简单的示例代码,演示如何使用该库:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using Microsoft.ML.OnnxRuntime;
using Microsoft.ML.OnnxRuntime.Tensors;
namespace ONNXNodeReader
{
class Program
{
static void Main(string[] args)
{
// Load the ONNX model
var session = new InferenceSession("model.onnx");
// Get the input and output node names
var inputName = session.InputMetadata.Keys.First();
var outputName = session.OutputMetadata.Keys.First();
// Create input tensor
var input = new DenseTensor<float>(new[] {1, 3, 224, 224});
// Fill input tensor with data
FillTensorWithRandomData(input);
// Run the model and get the output
var inputs = new List<NamedOnnxValue> {NamedOnnxValue.CreateFromTensor(inputName, input)};
var output = session.Run(inputs).First().AsTensor<float>();
// Print the output
Console.WriteLine($"Output shape: {string.Join(",", output.Shape)}");
Console.WriteLine($"Output data: {string.Join(",", output.ToArray())}");
}
static void FillTensorWithRandomData<T>(DenseTensor<T> tensor)
{
var random = new Random();
for (int i = 0; i < tensor.Length; i++)
{
tensor[i] = (T)Convert.ChangeType(random.NextDouble(), typeof(T));
}
}
}
}
```
在这个示例中,我们首先加载ONNX模型,然后获取输入和输出节点的名称。接下来,我们创建一个输入张量并将其填充到随机数据。最后,我们运行模型并打印输出。注意,这个示例只是一个简单的演示,实际上你可能需要根据你的具体场景进行修改。
希望这个示例可以帮助你开始在C#中读取ONNX节点数据。
阅读全文
相关推荐









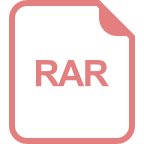




