go语言实现上传下载功能
时间: 2024-03-24 13:31:07 浏览: 24
以下是一个简单的Go语言实现文件上传和下载的示例代码:
上传文件:
```
func handleUpload(w http.ResponseWriter, r *http.Request) {
if r.Method != "POST" {
http.Error(w, "Method not allowed", http.StatusMethodNotAllowed)
return
}
file, _, err := r.FormFile("file")
if err != nil {
http.Error(w, err.Error(), http.StatusBadRequest)
return
}
defer file.Close()
// 将文件保存到本地
f, err := os.OpenFile("uploaded_file", os.O_WRONLY|os.O_CREATE, 0666)
if err != nil {
http.Error(w, err.Error(), http.StatusInternalServerError)
return
}
defer f.Close()
io.Copy(f, file)
w.WriteHeader(http.StatusOK)
}
```
下载文件:
```
func handleDownload(w http.ResponseWriter, r *http.Request) {
if r.Method != "GET" {
http.Error(w, "Method not allowed", http.StatusMethodNotAllowed)
return
}
f, err := os.Open("uploaded_file")
if err != nil {
http.Error(w, err.Error(), http.StatusInternalServerError)
return
}
defer f.Close()
// 设置响应头,告诉浏览器文件类型和文件名
w.Header().Set("Content-Type", "application/octet-stream")
w.Header().Set("Content-Disposition", fmt.Sprintf("attachment; filename=\"%s\"", "uploaded_file"))
io.Copy(w, f)
}
```
在main函数中,可以将这两个函数绑定到HTTP路由上:
```
func main() {
http.HandleFunc("/upload", handleUpload)
http.HandleFunc("/download", handleDownload)
http.ListenAndServe(":8080", nil)
}
```
现在,访问http://localhost:8080/upload可以上传文件,访问http://localhost:8080/download可以下载刚刚上传的文件。
相关推荐
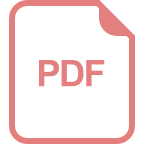
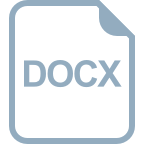
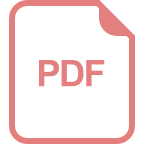
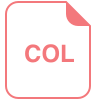
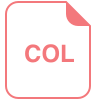
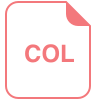
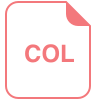
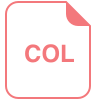









