用Java的do-while语句写一个从1!到10!的阶乘和
时间: 2023-05-31 20:03:14 浏览: 222
```
public class FactorialSum {
public static void main(String[] args) {
int i = 1;
int factorial = 1;
int sum = 0;
do {
factorial *= i;
sum += factorial;
i++;
} while (i <= 10);
System.out.println("1! + 2! + 3! + ... + 10! = " + sum);
}
}
```
相关问题
在java中用do-while语句计算1到10的阶乘之和
在Java中,我们可以使用do-while循环结合递归来计算从1到10的阶乘之和。首先,我们需要创建一个函数来计算阶乘,然后在do-while循环中不断累加阶乘结果,直到达到11(因为阶乘是从1开始计数的)。这里是一个示例:
```java
public class FactorialSum {
private static int factorial(int n) {
if (n == 0 || n == 1) {
return 1;
} else {
return n * factorial(n - 1);
}
}
public static void main(String[] args) {
int sum = 0;
int i = 1;
do {
sum += factorial(i);
i++;
} while (i <= 10);
System.out.println("1到10的阶乘之和是: " + sum);
}
}
```
在这个程序中,`factorial`函数通过递归实现了阶乘计算,而do-while循环则用于控制迭代次数,并将每个阶乘值累加到`sum`变量上。
编写程序。分别用while语句、do-while语句和for语句编写一个求阶乘N!的Java程序。
好的,这是一个计算阶乘的问题,可以使用while、do-while和for循环进行编写,以下是示例Java代码:
// 使用 while 循环求阶乘
public static int factorialWithWhile(int n) {
int result = 1;
while (n > 0) {
result *= n;
n--;
}
return result;
}
// 使用 do-while 循环求阶乘
public static int factorialWithDoWhile(int n) {
int result = 1;
do {
result *= n;
n--;
} while (n > 0);
return result;
}
// 使用 for 循环求阶乘
public static int factorialWithFor(int n) {
int result = 1;
for (int i = 1; i <= n; i++) {
result *= i;
}
return result;
}
以上三个方法分别用了 while、do-while和for循环来求n的阶乘,可以根据需要进行选择。
阅读全文
相关推荐

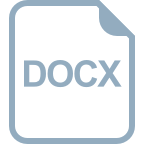











