vue2 项目,有一个项目详情页,详情页内由上到下有5个部分进行排列,要求使用自定义指令v-scroll并封装一个固定于右侧竖向排列的快速定位标签,实现点击标签页面滚动到相应的模块,并且页面上下滚动时候标签会联动高亮的功能,请告诉我实现这个功能的逻辑及代码
时间: 2024-02-11 13:09:47 浏览: 18
实现这个功能的逻辑如下:
1. 在Vue项目中定义一个v-scroll自定义指令,用于实现页面的滚动效果。
2. 在详情页中添加一个固定于右侧竖向排列的快速定位标签,用于点击跳转到相应的模块。
3. 监听页面的滚动事件,根据滚动的位置更新标签的高亮状态。
4. 根据点击的标签获取对应的模块的位置,使用JavaScript的scrollIntoView()方法实现页面滚动到指定模块的位置。
下面是实现这个功能的代码示例:
1. 在项目中定义v-scroll自定义指令
```
//main.js
Vue.directive('scroll', {
inserted: function (el, binding) {
let f = function (evt) {
if (binding.value(evt, el)) {
window.removeEventListener('scroll', f)
}
}
window.addEventListener('scroll', f)
}
})
```
2. 在详情页中添加快速定位标签
```
<template>
<div class="wrapper">
<div class="nav">
<ul>
<li v-for="(item, index) in list" :key="index" :class="{active: activeIndex===index}" @click="scrollTo(index)">{{item.title}}</li>
</ul>
</div>
<div class="content">
<div class="item" v-for="(item, index) in list" :key="index">
<h2>{{item.title}}</h2>
<p>{{item.content}}</p>
</div>
</div>
</div>
</template>
<script>
export default {
name: 'DetailPage',
data () {
return {
list: [
{
title: 'Section 1',
content: 'Content of section 1'
},
{
title: 'Section 2',
content: 'Content of section 2'
},
{
title: 'Section 3',
content: 'Content of section 3'
},
{
title: 'Section 4',
content: 'Content of section 4'
},
{
title: 'Section 5',
content: 'Content of section 5'
}
],
activeIndex: 0
}
},
methods: {
scrollTo (index) {
// 获取对应模块的位置
let target = this.$el.querySelectorAll('.item')[index]
let offsetTop = target.offsetTop
// 页面滚动到指定模块的位置
window.scrollTo({
top: offsetTop,
behavior: 'smooth'
})
},
handleScroll (evt, el) {
// 监听页面滚动事件,根据滚动的位置更新标签的高亮状态
let scrollTop = window.scrollY
let items = this.$el.querySelectorAll('.item')
for (let i = 0; i < items.length; i++) {
let item = items[i]
let offsetTop = item.offsetTop
if (offsetTop <= scrollTop) {
this.activeIndex = i
}
}
return false
}
},
directives: {
// 使用v-scroll自定义指令实现页面滚动效果
scroll: {
inserted: function (el, binding) {
let f = function (evt) {
if (binding.value(evt, el)) {
window.removeEventListener('scroll', f)
}
}
window.addEventListener('scroll', f)
}
}
}
}
</script>
<style>
.wrapper {
display: flex;
}
.nav {
flex: 0 0 200px;
border-right: 1px solid #ccc;
}
.nav ul {
list-style: none;
padding: 0;
margin: 0;
}
.nav li {
cursor: pointer;
padding: 10px;
border-bottom: 1px solid #ccc;
}
.nav li.active {
color: red;
}
.content {
flex: 1;
}
.item {
margin: 20px 0;
}
</style>
```
在上面的代码中,我们在详情页中添加了一个快速定位标签,使用v-for指令渲染列表,并且使用:class绑定样式来实现标签的高亮效果。同时,我们还监听了标签的点击事件,当用户点击标签时,我们通过scrollTo()方法将页面滚动到对应模块的位置。
我们还定义了一个v-scroll自定义指令,用于监听页面的滚动事件,并在滚动时更新标签的高亮状态。在该指令中,我们使用了window.scrollTo()方法实现页面的滚动效果。
最后,我们使用了一些CSS样式来实现页面的布局和样式。
希望这个示例能够帮助你实现你的需求。
相关推荐
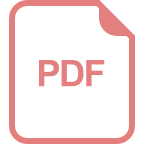
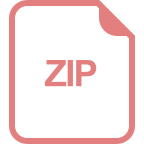
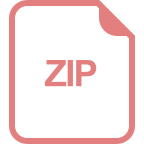














