pytorch lsem
时间: 2023-10-28 22:57:22 浏览: 51
PyTorch is a popular deep learning framework that provides various modules and tools for building and training neural networks. The LSTM module in PyTorch is used for implementing Long Short-Term Memory (LSTM) networks, which are a type of recurrent neural network (RNN).
To use LSTM in PyTorch, you first need to import the necessary modules:
```python
import torch
import torch.nn as nn
```
Then, you can define an LSTM network using the `nn.LSTM` class. Here's a simple example of how to define an LSTM network with one input layer, one LSTM layer, and one output layer:
```python
class LSTMModel(nn.Module):
def __init__(self, input_size, hidden_size, num_layers, output_size):
super(LSTMModel, self).__init__()
self.hidden_size = hidden_size
self.num_layers = num_layers
self.lstm = nn.LSTM(input_size, hidden_size, num_layers, batch_first=True)
self.fc = nn.Linear(hidden_size, output_size)
def forward(self, x):
h0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(x.device)
c0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(x.device)
out, _ = self.lstm(x, (h0, c0))
out = self.fc(out[:, -1, :])
return out
```
In this example, `input_size` represents the number of features in the input data, `hidden_size` is the number of features in the hidden state of the LSTM, `num_layers` is the number of recurrent layers, and `output_size` is the number of output features.
You can then create an instance of the `LSTMModel` class and use it for training and inference:
```python
input_size = 10
hidden_size = 20
num_layers = 2
output_size = 1
model = LSTMModel(input_size, hidden_size,
相关推荐
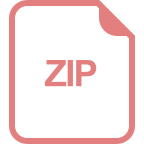
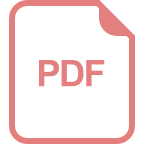
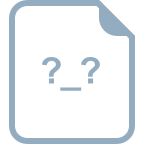
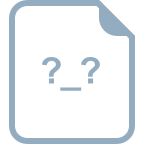
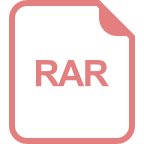
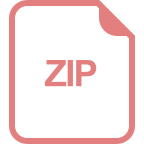
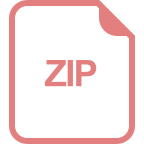
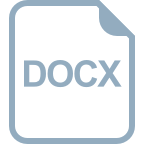