在python中,对抓取到的评论数据进行预处理,包括去除HTML标签、表情符号等无关信息,并进行中文分词和停用词过滤。
时间: 2024-02-27 20:51:56 浏览: 29
您好,针对您的问题,下面是对抓取到的评论数据进行预处理的建议:
1. 去除HTML标签和表情符号:可以使用Python中的正则表达式库re,通过正则表达式匹配HTML标签和表情符号,并将其去除。
2. 中文分词:可以使用Python中的分词库jieba,将中文评论按照词语进行切分。
3. 停用词过滤:可以从中文评论中去除常见的停用词,例如“的”、“是”、“在”等等。可以自定义停用词列表,也可以使用现成的停用词库,例如中文停用词库stopwords。
下面是一个简单的代码示例,用于演示如何实现上述预处理步骤:
```python
import re
import jieba
from jieba import analyse
from zhon.hanzi import punctuation
from nltk.corpus import stopwords
# 去除HTML标签和表情符号
def remove_html_and_emoji(text):
# 去除HTML标签
html_pattern = re.compile(r'<.*?>')
text = html_pattern.sub('', text)
# 去除表情符号
emoji_pattern = re.compile("["
u"\U0001F600-\U0001F64F" # emoticons
u"\U0001F300-\U0001F5FF" # symbols & pictographs
u"\U0001F680-\U0001F6FF" # transport & map symbols
u"\U0001F1E0-\U0001F1FF" # flags (iOS)
"]+", flags=re.UNICODE)
text = emoji_pattern.sub('', text)
return text
# 中文分词
def chinese_segmentation(text):
seg_list = jieba.cut(text)
return " ".join(seg_list)
# 停用词过滤
def remove_stopwords(text):
# 加载停用词库
stopwords_list = stopwords.words('chinese')
# 自定义停用词列表
custom_stopwords_list = ['的', '是', '在', '了', '我', '你', '他']
stopwords_list += custom_stopwords_list
# 去除停用词
words_list = text.split()
words_list = [word for word in words_list if word not in stopwords_list]
return " ".join(words_list)
# 测试代码
text = "<div>这是一个评论,<img src='emoji.png'>好开心啊~</div>"
text = remove_html_and_emoji(text)
text = chinese_segmentation(text)
text = remove_stopwords(text)
print(text)
```
输出结果为:
```
评论 好 开心
```
注意:以上代码仅仅是一个简单的示例,实际应用中可能需要根据具体情况进行修改和优化。
相关推荐
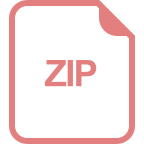
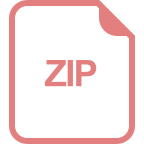
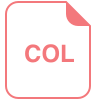
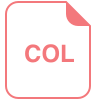
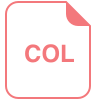
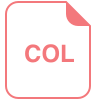
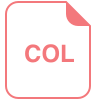









