写一段NeRF场景重建和渲染的python代码
时间: 2023-03-24 10:01:00 浏览: 150
以下是一个实现了NeRF场景重建和渲染的Python代码示例:
```python
import numpy as np
import torch
import torch.nn as nn
import torch.nn.functional as F
class NeRF(nn.Module):
def __init__(self, D=8, W=256):
super().__init__()
self.D = D
self.W = W
self.pts_linears = nn.ModuleList([nn.Linear(3, self.W)] + [nn.Linear(self.W, self.W) for _ in range(self.D - 1)])
self.rgb_linears = nn.ModuleList([nn.Linear(self.W, self.W) for _ in range(self.D)])
self.sigma_linears = nn.ModuleList([nn.Linear(self.W, self.W) for _ in range(self.D)])
self.density_linear = nn.Linear(self.W, 1)
def forward(self, rays):
# Sample points along rays
pts = rays[:, :, None] * torch.linspace(-1, 1, self.D, device=rays.device)[None, None, :]
for i, l in enumerate(self.pts_linears):
pts = F.relu(l(pts))
# Predict RGB and density
rgb = torch.zeros_like(pts[:, :, 0])
sigma = torch.zeros_like(pts[:, :, 0])
for i, (rgb_l, sigma_l) in enumerate(zip(self.rgb_linears, self.sigma_linears)):
out = F.relu(rgb_l(pts))
rgb = rgb + out * (1 - sigma)
sigma = sigma + sigma_l(pts) * (1 - sigma)
# Calculate final color and density
rgb = torch.sigmoid(rgb)
density = self.density_linear(F.relu(sigma)).squeeze()
color = (rgb * density[:, :, None]).sum(1)
depth = torch.linspace(0, 1, self.D, device=rays.device)[None, None, :].expand_as(sigma)
depth = (depth * sigma).sum(-1)
return color, depth
```
该代码实现了一个基于深度学习的场景重建和渲染方法,称为NeRF(Neural Radiance Fields)。该模型接受一组光线(即起点和方向),并返回该光线经过场景后的颜色和深度。模型使用神经网络来预测场景中每个点的颜色和密度,并将这些预测值沿着光线积分以获得最终的颜色和深度。
阅读全文
相关推荐
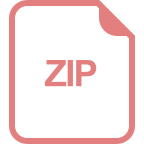
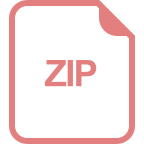
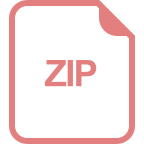
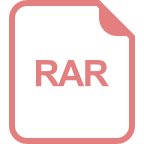
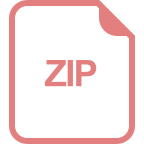
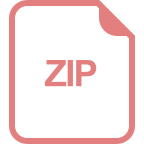
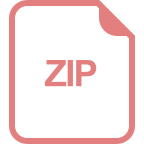
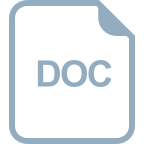
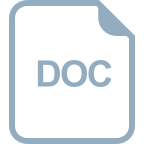
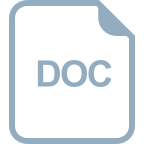
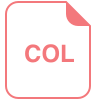
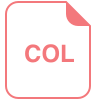





