我想要一个Java的冒泡算法
时间: 2023-09-10 21:05:35 浏览: 81
### 回答1:
。今天是2020年8月7日。
我可以提供一段Java的冒泡算法的代码:public static void bubbleSort(int[] array) { int n = array.length; int temp = 0; for(int i=0; i < n; i++){ for(int j=1; j < (n-i); j++){ if(array[j-1] > array[j]){ temp = array[j-1]; array[j-1] = array[j]; array[j] = temp; } } } }
### 回答2:
冒泡排序算法是一种简单但效率较低的排序算法。下面是一个用Java编写的冒泡排序算法示例:
```java
public class BubbleSort {
public static void bubbleSort(int[] arr) {
int n = arr.length;
for (int i = 0; i < n-1; i++) {
for (int j = 0; j < n-i-1; j++) {
if (arr[j] > arr[j+1]) {
// 交换arr[j]和arr[j+1]
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
}
public static void main(String[] args) {
int[] arr = {64, 34, 25, 12, 22, 11, 90};
bubbleSort(arr);
System.out.println("排序后的数组:");
for (int num : arr) {
System.out.print(num + " ");
}
}
}
```
在上面的例子中,我们定义了一个`bubbleSort()`方法,该方法接收一个整数数组作为参数,并使用冒泡排序算法对数组进行排序。内嵌的两个循环会遍历数组,并比较相邻元素的大小,如果发现前面的元素大于后面的元素,则交换它们。最终得到的结果是一个按升序排列的数组。
在`main()`方法中,我们创建了一个整数数组,并调用`bubbleSort()`方法对其进行排序。然后使用循环打印排序后的数组。
需要注意的是,冒泡排序算法的时间复杂度为O(n^2),在处理大规模数据时效率较低,但对于小规模数据或近乎有序的数据,冒泡排序算法仍然是一种简单且可行的选择。
### 回答3:
冒泡排序是一种简单且经典的排序算法,它可以通过比较相邻元素的大小来对一个数组进行排序。以下是一个使用Java实现的冒泡排序算法。
```java
public class BubbleSort {
public static void main(String[] args) {
int[] arr = {5, 2, 8, 1, 9};
bubbleSort(arr);
System.out.println("排序后的数组:");
for (int num : arr) {
System.out.print(num + " ");
}
}
public static void bubbleSort(int[] arr) {
int n = arr.length;
boolean swapped;
for (int i = 0; i < n - 1; i++) {
swapped = false;
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// 交换两个元素
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
swapped = true;
}
}
// 如果没有发生交换,说明数组已经有序,可以提前结束排序
if (!swapped) {
break;
}
}
}
}
```
以上代码演示了如何使用冒泡排序算法对一个整型数组进行排序。在主函数中,我们定义一个数组并调用`bubbleSort`函数进行排序。内部实现中,我们使用两层循环遍历数组并进行比较,如果相邻元素的顺序不正确,则进行交换。每经过一轮循环,最大的元素会被移动到数组的末尾。最后,我们输出排序后的数组。
冒泡排序的时间复杂度为O(n^2),其中n是数组的大小。虽然冒泡排序算法简单,但对于大型数据集合来说效率较低,不适用于处理大规模数据。
阅读全文
相关推荐
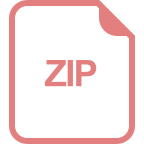
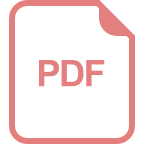
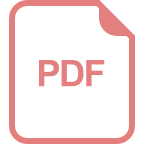
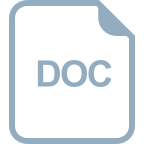
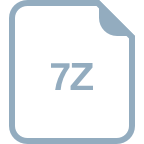
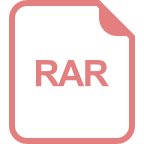
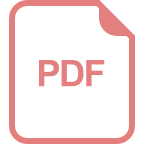
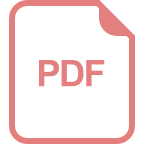
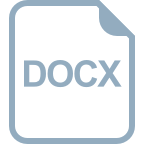
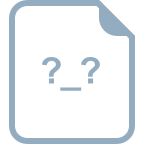
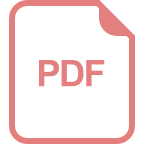