如何使用machine learning 排出边框干扰识别数据。做一个python程序,输出代码
时间: 2024-10-15 12:11:54 浏览: 14
在使用机器学习排出边框干扰以识别图像数据时,通常会采用计算机视觉技术结合深度学习模型。这里我们将通过Python和流行的库如TensorFlow或PyTorch来实现一个简单的例子。首先,我们需要准备数据集、预处理步骤、模型训练以及最终的预测。
**1. 准备环境和导入必要的库**
```python
import numpy as np
import matplotlib.pyplot as plt
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Dropout, Flatten, Dense
from tensorflow.keras.preprocessing.image import ImageDataGenerator
```
**2. 数据预处理**
创建`ImageDataGenerator`对象,用于对图像进行归一化、随机旋转等操作,减少边框干扰的影响。
```python
data_generator = ImageDataGenerator(rescale=1./255, rotation_range=40, width_shift_range=0.2, height_shift_range=0.2)
train_data = data_generator.flow_from_directory('path/to/train/directory', target_size=(img_width, img_height), batch_size=batch_size, class_mode='binary')
validation_data = data_generator.flow_from_directory('path/to/validation/directory', target_size=(img_width, img_height), batch_size=batch_size, class_mode='binary')
```
**3. 构建卷积神经网络(CNN)模型**
```python
model = Sequential([
Conv2D(32, (3, 3), activation='relu', input_shape=(img_width, img_height, 3)),
MaxPooling2D((2, 2)),
Conv2D(64, (3, 3), activation='relu'),
MaxPooling2D((2, 2)),
Conv2D(128, (3, 3), activation='relu'),
MaxPooling2D((2, 2)),
Flatten(),
Dense(128, activation='relu'),
Dropout(0.5),
Dense(1, activation='sigmoid')
])
```
**4. 编译和训练模型**
```python
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
history = model.fit(train_data, epochs=num_epochs, validation_data=validation_data)
```
**5. 预测新数据**
```python
# 加载测试图片并进行预处理
test_image = load_and_preprocess_test_image('path/to/test/image.jpg')
# 进行预测
prediction = model.predict(test_image.reshape(-1, img_width, img_height, 3))
```
**
阅读全文
相关推荐
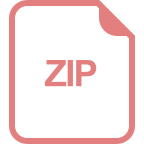
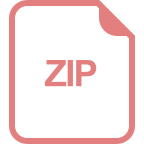
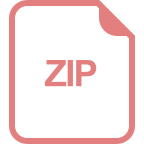
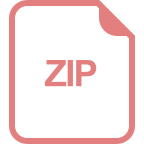
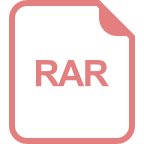
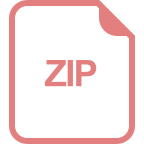
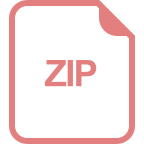
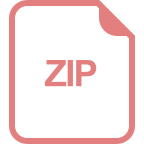
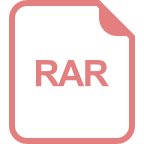
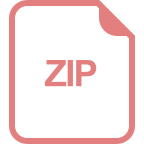
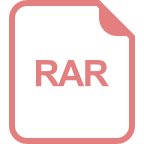