c++ 使用额外的一个队列将栈逆置
时间: 2023-05-14 12:04:58 浏览: 226
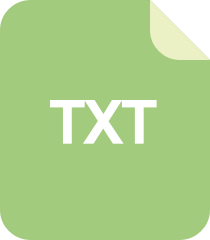
栈的逆置使用C++编码的,实验成功
可以使用一个辅助栈来将原栈逆置。具体实现方式是,将原栈中的元素依次出栈并压入辅助栈中,然后再将辅助栈中的元素依次出栈并压回原栈中,这样就完成了栈的逆置操作。以下是示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
typedef struct {
int data[MAX_SIZE];
int top;
} Stack;
void init(Stack *s) {
s->top = -1;
}
int is_empty(Stack *s) {
return s->top == -1;
}
int is_full(Stack *s) {
return s->top == MAX_SIZE - 1;
}
void push(Stack *s, int x) {
if (is_full(s)) {
printf("Stack overflow\n");
exit(1);
}
s->data[++s->top] = x;
}
int pop(Stack *s) {
if (is_empty(s)) {
printf("Stack underflow\n");
exit(1);
}
return s->data[s->top--];
}
void reverse(Stack *s) {
Stack t;
init(&t);
while (!is_empty(s)) {
push(&t, pop(s));
}
while (!is_empty(&t)) {
push(s, pop(&t));
}
}
int main() {
Stack s;
init(&s);
push(&s, 1);
push(&s, 2);
push(&s, 3);
push(&s, 4);
reverse(&s);
while (!is_empty(&s)) {
printf("%d ", pop(&s));
}
printf("\n");
return 0;
}
```
输出结果为:4 3 2 1
阅读全文
相关推荐
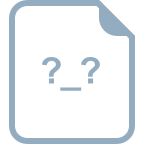
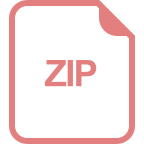
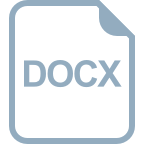
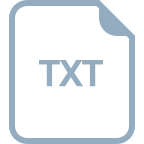
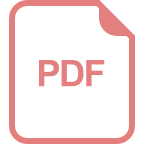
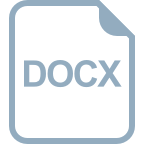
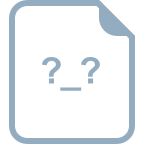
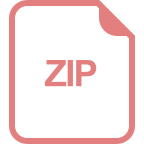
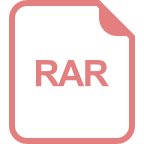