【问题描述】输入某个学生的信息(含学号,姓名,年龄,六门课程分数-高数/英语/c语言/工程制图/大学物理/信息论,它们的课程积分分别为4/3/4/3/3/3),计算课程总积分。 【输入形式】 学号 姓名 年龄 课程分数 【输出形式】 学号 姓名 年龄 课程总积分 【样例输入】 18201819 zhangone 20 90 57 81 85 79 91 【样例输出】 18201819 zhangone 20 17用c语言
时间: 2023-06-25 12:04:09 浏览: 56
下面是一个Python的实现,可以帮助你理解这道题目:
```python
score_weight = [4, 3, 4, 3, 3, 3] # 课程积分权重
# 输入学生信息
info = input().split()
student_id, name, age = info[0], info[1], info[2]
scores = list(map(int, info[3:]))
# 计算总积分
total_score = sum([score * weight for score, weight in zip(scores, score_weight)])
# 输出结果
print(f"{student_id} {name} {age} {total_score}")
```
我们首先定义了一个列表 `score_weight` 表示每门课程的积分权重,然后通过 `input()` 函数获取用户输入的学生信息。接着,我们使用 `split()` 函数将输入的字符串分割为一个列表 `info`,其中第一个元素是学号,第二个元素是姓名,第三个元素是年龄,剩余的元素是六门课程的分数。
然后,我们使用 `map()` 函数将剩余的元素从字符串转换为整数,并使用 `zip()` 函数将每门课程的分数和对应的积分权重组合在一起。最后,我们使用列表推导式计算每门课程的积分贡献,再用 `sum()` 函数求和得到总积分。
最后,我们使用 `print()` 函数输出结果,格式化字符串中的变量用 `{}` 表示。
相关问题
输入某个学生的信息(含学号,姓名,年龄,六门课程分数-高数/英语/c语言/工程制图/大学物理/信息论,它们的课程积分分别为4/3/4/3/3/3),计算课程总积分。c语言结构体代码
以下是计算课程总积分的C语言结构体代码示例:
```c
#include <stdio.h>
struct student {
int id; // 学号
char name[20]; // 姓名
int age; // 年龄
int score_math; // 高数分数
int score_english; // 英语分数
int score_c; // C语言分数
int score_engineering; // 工程制图分数
int score_physics; // 大学物理分数
int score_information; // 信息论分数
};
int main() {
struct student stu;
int total_score;
// 输入学生信息
printf("请输入学生信息:\n");
printf("学号:");
scanf("%d", &stu.id);
printf("姓名:");
scanf("%s", stu.name);
printf("年龄:");
scanf("%d", &stu.age);
printf("高数分数:");
scanf("%d", &stu.score_math);
printf("英语分数:");
scanf("%d", &stu.score_english);
printf("C语言分数:");
scanf("%d", &stu.score_c);
printf("工程制图分数:");
scanf("%d", &stu.score_engineering);
printf("大学物理分数:");
scanf("%d", &stu.score_physics);
printf("信息论分数:");
scanf("%d", &stu.score_information);
// 计算课程总积分
total_score = 4 * stu.score_math + 3 * stu.score_english + 4 * stu.score_c + 3 * stu.score_engineering + 3 * stu.score_physics + 3 * stu.score_information;
// 输出结果
printf("课程总积分为:%d\n", total_score);
return 0;
}
```
注意:以上代码仅提供参考,实际应用中可能需要根据具体需求进行修改。
c语言:输入某个学生的信息(含学号,姓名,年龄,六门课程分数-高数/英语/c语言/工程制图/大学物理/信息论,它们的课程积分分别为4/3/4/3/3/3),计算课程总积分。 【输入形式】 学号 姓名 年龄 课程分数 【输出形式】 学号 姓名 年龄 课程总积分
以下是代码实现:
```c
#include <stdio.h>
int main() {
int id, age;
char name[20];
float math_score, eng_score, c_score, graph_score, physics_score, info_score;
float total_score;
printf("请输入学生信息(学号 姓名 年龄 高数英语c语言工程制图大学物理信息论分数):\n");
scanf("%d %s %d %f %f %f %f %f %f", &id, name, &age, &math_score, &eng_score, &c_score, &graph_score, &physics_score, &info_score);
total_score = math_score*4 + eng_score*3 + c_score*4 + graph_score*3 + physics_score*3 + info_score*3;
printf("学号:%d\n姓名:%s\n年龄:%d\n课程总积分:%.2f\n", id, name, age, total_score);
return 0;
}
```
这段代码实现了输入某个学生的信息(包括学号、姓名、年龄、六门课程分数)并计算课程总积分。通过 `scanf` 函数获取输入信息,然后将每个科目的分数乘以对应的课程积分后相加得到总积分,最后输出学生的信息和课程总积分。
相关推荐
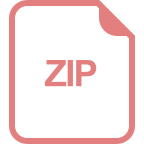
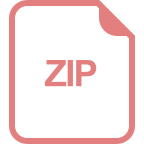


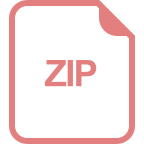
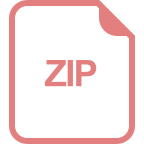
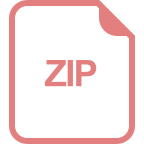
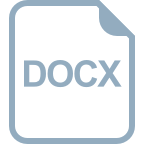
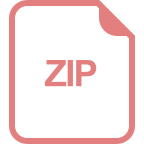
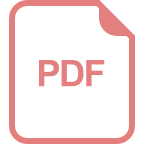
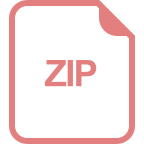
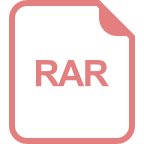
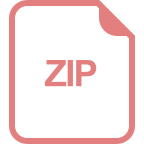
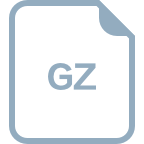
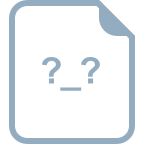
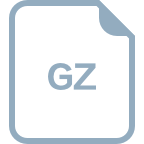