编程实现三种颜色空间转换的算法,实现不同颜色空间的自动转换matlab
时间: 2023-11-28 17:47:53 浏览: 126
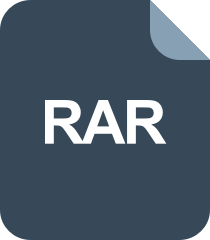
基于Matlab实现图像颜色空间相互转换(源码+数据).rar

以下是三种颜色空间转换的算法和Matlab代码实现:
1. RGB到HSV的转换算法:
HSV色彩空间将颜色描述为三元素:色调(Hue)、饱和度(Saturation)和亮度(Value)。该转换算法的实现如下:
```matlab
function [H,S,V] = RGB2HSV(R,G,B)
% RGB2HSV - Convert Red-Green-Blue Color value to Hue-Saturation-Value
% Usage:
% [H,S,V] = RGB2HSV(R,G,B)
%
% RGB is an m x 3 matrix with each row representing one color.
% H,S,V are m x 1 vectors representing Hue, Saturation, Value respectively.
%
% Author: Shanqing Cai (shanqing.cai@gmail.com)
% Date: 2010-09-17
if nargin < 3
error('RGB2HSV requires 3 input arguments.');
end
R = double(R);
G = double(G);
B = double(B);
% Normalize RGB values
R = R / 255;
G = G / 255;
B = B / 255;
% Find the maximum and minimum values of RGB
Max = max(max(R,G),B);
Min = min(min(R,G),B);
% Calculate the Value
V = Max;
Delta = Max - Min;
% Calculate the Saturation
if Max ~= 0
S = Delta ./ Max;
else
S = 0;
end
% Calculate the Hue
if Delta ~= 0
if Max == R
H = 60 * mod((G-B)./Delta,6);
elseif Max == G
H = 60 * ((B-R)./Delta + 2);
elseif Max == B
H = 60 * ((R-G)./Delta + 4);
end
else
H = 0;
end
H(H<0) = H(H<0) + 360;
% Convert Hue to range [0,1]
H = H / 360;
```
2. RGB到YCbCr的转换算法:
YCbCr色彩空间是由亮度(Y)和色度(Cb和Cr)分量组成的,可以更好地表示彩色图像。该转换算法的实现如下:
```matlab
function [Y,Cb,Cr] = RGB2YCbCr(R,G,B)
% RGB2YCbCr - Convert Red-Green-Blue Color value to YCbCr
% Usage:
% [Y,Cb,Cr] = RGB2YCbCr(R,G,B)
%
% RGB is an m x 3 matrix with each row representing one color.
% Y,Cb,Cr are m x 1 vectors representing Y, Cb, Cr respectively.
%
% Author: Shanqing Cai (shanqing.cai@gmail.com)
% Date: 2010-09-17
if nargin < 3
error('RGB2YCbCr requires 3 input arguments.');
end
R = double(R);
G = double(G);
B = double(B);
% Normalize RGB values
R = R / 255;
G = G / 255;
B = B / 255;
% Convert RGB to YCbCr
Y = 0.299 * R + 0.587 * G + 0.114 * B;
Cb = -0.168736 * R - 0.331264 * G + 0.5 * B + 128;
Cr = 0.5 * R - 0.418688 * G - 0.081312 * B + 128;
```
3. RGB到Lab的转换算法:
Lab色彩空间是由亮度(L)和色度(a和b)分量组成的,可以更好地表示颜色的人类感知。该转换算法的实现如下:
```matlab
function [L,a,b] = RGB2Lab(R,G,B)
% RGB2Lab - Convert Red-Green-Blue Color value to Lab
% Usage:
% [L,a,b] = RGB2Lab(R,G,B)
%
% RGB is an m x 3 matrix with each row representing one color.
% L,a,b are m x 1 vectors representing L*, a* and b* respectively.
%
% Author: Shanqing Cai (shanqing.cai@gmail.com)
% Date: 2010-09-17
if nargin < 3
error('RGB2Lab requires 3 input arguments.');
end
R = double(R);
G = double(G);
B = double(B);
% Normalize RGB values
R = R / 255;
G = G / 255;
B = B / 255;
% Convert RGB to XYZ
X = 0.412453*R + 0.357580*G + 0.180423*B;
Y = 0.212671*R + 0.715160*G + 0.072169*B;
Z = 0.019334*R + 0.119193*G + 0.950227*B;
% Convert XYZ to Lab
Xn = 95.047;
Yn = 100.000;
Zn = 108.883;
L = 116 * f(Y/Yn) - 16;
a = 500 * (f(X/Xn) - f(Y/Yn));
b = 200 * (f(Y/Yn) - f(Z/Zn));
function t = f(t)
t(t > 0.008856) = t(t > 0.008856).^(1/3);
t(t <= 0.008856) = 7.787*t(t <= 0.008856) + 16/116;
end
```
在Matlab中,可以使用以下命令来进行颜色空间转换:
```matlab
% RGB to HSV
[H,S,V] = rgb2hsv(R,G,B);
% RGB to YCbCr
[Y,Cb,Cr] = rgb2ycbcr(R,G,B);
% RGB to Lab
[L,a,b] = rgb2lab(R,G,B);
```
其中,R、G、B分别表示红、绿、蓝三个颜色通道的值。
阅读全文
相关推荐
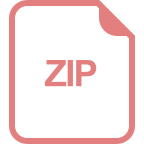
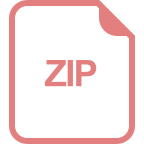
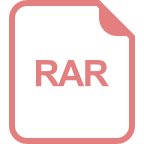
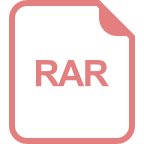
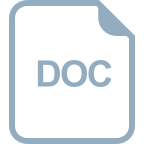
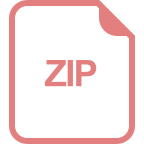
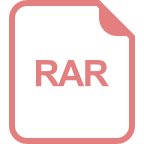
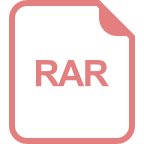
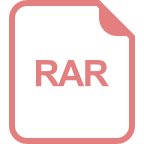
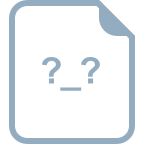
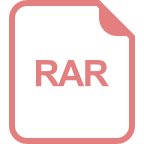
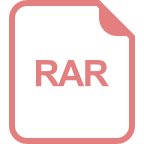
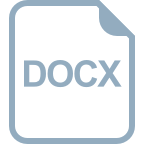
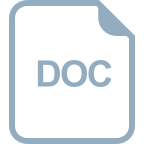
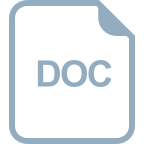
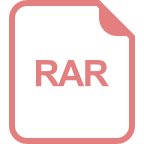
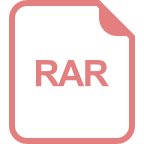