java模拟工人挑苹果
时间: 2023-10-11 12:18:46 浏览: 126
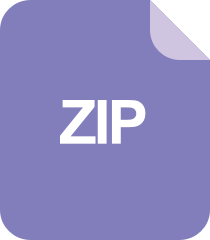
模拟苹果的ishut

好的,我会尽力回答你的问题。在模拟工人挑苹果的过程中,我们需要考虑以下几个方面:
1. 苹果的属性:大小、颜色、位置等等。
2. 工人的能力:挑苹果的速度、准确度等等。
3. 挑选策略:工人如何选择哪些苹果挑选,如何避免重复挑选等等。
基于以上几个方面,我们可以设计出一种简单的Java程序来模拟工人挑苹果的过程,以下是一个示例代码:
```
import java.util.ArrayList;
import java.util.Random;
class Apple{
private int size;
private String color;
private int x, y;
public Apple(int size, String color, int x, int y){
this.size = size;
this.color = color;
this.x = x;
this.y = y;
}
public int getSize(){
return size;
}
public String getColor(){
return color;
}
public int getX(){
return x;
}
public int getY(){
return y;
}
}
class Worker{
private int speed;
private int accuracy;
public Worker(int speed, int accuracy){
this.speed = speed;
this.accuracy = accuracy;
}
public int getSpeed(){
return speed;
}
public int getAccuracy(){
return accuracy;
}
public void pickApple(ArrayList<Apple> apples){
Random random = new Random();
for(Apple apple : apples){
if(random.nextInt(100) < accuracy){
System.out.println("Worker picked an apple: " + apple.getColor() + " apple, size " + apple.getSize() + ", position (" + apple.getX() + ", " + apple.getY() + ")");
apples.remove(apple);
break;
}
}
}
}
public class Main{
public static void main(String[] args){
ArrayList<Apple> apples = new ArrayList<>();
apples.add(new Apple(2, "red", 10, 20));
apples.add(new Apple(3, "green", 30, 40));
apples.add(new Apple(1, "yellow", 50, 60));
Worker worker = new Worker(10, 80);
for(int i = 0; i < 5; i++){
System.out.println("Round " + (i+1) + ":");
worker.pickApple(apples);
}
}
}
```
在这个示例代码中,我们定义了一个Apple类和一个Worker类,分别表示苹果和工人。Apple类包含苹果的属性,Worker类包含工人的能力。我们在Main类中创建了若干个Apple对象,并创建了一个Worker对象。在Main类的主函数中,我们模拟了工人挑苹果的过程,输出了每一轮挑选的结果。Worker类中的pickApple方法模拟了工人挑选苹果的过程,其中使用了一个随机数来模拟工人的准确度。
这只是一个简单的示例代码,你可以根据自己的需求进行修改和扩展。希望能对你有所帮助!
阅读全文
相关推荐
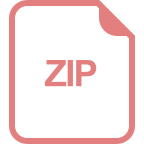
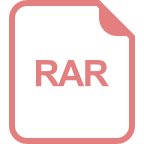
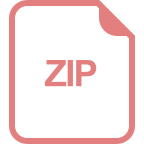
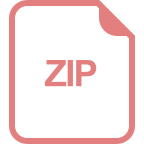
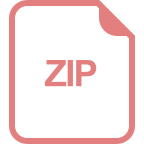
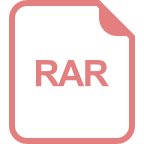
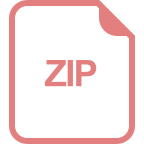
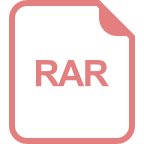
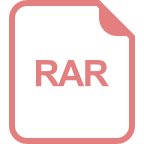
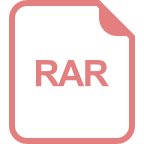
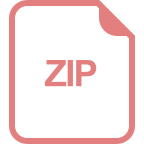
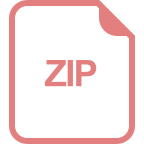