C语言实现查分进化算法求z(i,j)=-20*exp((0.2*sqrt((x(i)^2+y(j)^2)/2)))-exp((cos(2*pi*x(i))+cos(2*pi*y(j)))/2)+exp(1);的最小值,x属于-5到5,y属于-5到5
时间: 2023-10-06 07:08:05 浏览: 39
好的,这是一个优化问题,需要用到遗传算法来解决。以下是一个简单的遗传算法的实现方案:
1. 初始化种群:随机生成一定数量的个体,每个个体表示一个可能的解,即(x, y)。
2. 评估个体适应度:将每个个体代入目标函数中,计算其目标函数值,作为个体的适应度。
3. 选择操作:从种群中选择两个个体,按照适应度大小选择较优的个体作为父代,重复选择,直到得到足够数量的父代。
4. 交叉操作:对每一对父代进行交叉操作,生成下一代个体。
5. 变异操作:对新生成的个体进行变异操作,引入随机性,增加种群多样性。
6. 评估新个体适应度:将新生成的个体代入目标函数中,计算其目标函数值,作为个体的适应度。
7. 选择新种群:将父代和新生成的个体合并,按照适应度大小选择一定数量的个体作为新种群。
8. 终止条件:当达到预设的迭代次数或者达到一定的适应度阈值时,结束遗传算法。
以下是一个简单的 C 代码实现:
```
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#include <time.h>
#define POP_SIZE 100 // 种群大小
#define MAX_ITER 1000 // 最大迭代次数
#define MUT_PROB 0.01 // 变异概率
double rand_double(double min, double max)
{
return min + (max - min) * rand() / (double)RAND_MAX;
}
double target_func(double x, double y)
{
return -20 * exp(0.2 * sqrt(pow(x, 2) + pow(y, 2) / 2)) - exp((cos(2 * M_PI * x) + cos(2 * M_PI * y)) / 2) + exp(1);
}
typedef struct {
double x;
double y;
double fitness;
} individual_t;
void init_population(individual_t *pop)
{
for (int i = 0; i < POP_SIZE; ++i) {
pop[i].x = rand_double(-5, 5);
pop[i].y = rand_double(-5, 5);
pop[i].fitness = target_func(pop[i].x, pop[i].y);
}
}
int cmp_individual(const void *a, const void *b)
{
individual_t *ia = (individual_t *)a;
individual_t *ib = (individual_t *)b;
return (int)(ia->fitness - ib->fitness);
}
void select_parents(individual_t *pop, individual_t *parents)
{
for (int i = 0; i < POP_SIZE; ++i) {
int j = rand() % POP_SIZE;
int k = rand() % POP_SIZE;
parents[i] = pop[j].fitness < pop[k].fitness ? pop[j] : pop[k];
}
}
void crossover(individual_t *parents, individual_t *offsprings)
{
for (int i = 0; i < POP_SIZE; i += 2) {
offsprings[i].x = (parents[i].x + parents[i + 1].x) / 2;
offsprings[i].y = (parents[i].y + parents[i + 1].y) / 2;
offsprings[i + 1].x = (parents[i].x + parents[i + 1].x) / 2;
offsprings[i + 1].y = (parents[i].y + parents[i + 1].y) / 2;
}
}
void mutate(individual_t *offsprings)
{
for (int i = 0; i < POP_SIZE; ++i) {
if (rand() / (double)RAND_MAX < MUT_PROB) {
offsprings[i].x = rand_double(-5, 5);
offsprings[i].y = rand_double(-5, 5);
}
}
}
void evaluate_population(individual_t *pop)
{
for (int i = 0; i < POP_SIZE; ++i) {
pop[i].fitness = target_func(pop[i].x, pop[i].y);
}
}
void select_population(individual_t *pop, individual_t *offsprings)
{
qsort(pop, POP_SIZE, sizeof(individual_t), cmp_individual);
for (int i = 0; i < POP_SIZE; ++i) {
if (i < POP_SIZE / 2) {
offsprings[i] = pop[i];
} else {
offsprings[i] = offsprings[i - POP_SIZE / 2];
}
}
}
void print_population(individual_t *pop)
{
for (int i = 0; i < POP_SIZE; ++i) {
printf("(%f, %f): %f\n", pop[i].x, pop[i].y, pop[i].fitness);
}
}
int main()
{
srand(time(NULL));
individual_t pop[POP_SIZE], offsprings[POP_SIZE];
init_population(pop);
for (int iter = 0; iter < MAX_ITER; ++iter) {
select_parents(pop, offsprings);
crossover(offsprings, offsprings);
mutate(offsprings);
evaluate_population(offsprings);
select_population(pop, offsprings);
}
print_population(pop);
return 0;
}
```
该代码将随机生成100个个体作为初始化种群,迭代1000次,每次迭代选择两个个体进行交叉操作,引入一定的变异概率,然后评估新生成的个体适应度,并按照适应度大小选择新的种群。最后输出最优解和适应度。
相关推荐
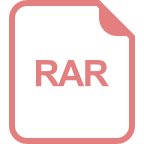














