1、 请编写一个简单的使用FIFO进行IPC的客户端/服务器应用程序。服务器提供的(简单)服务是向每个发送请求的客户端赋一个唯一的顺序数字。写出在Linux下编写
时间: 2023-02-22 07:47:37 浏览: 116
FIFO(先进先出)是一种进程间通信(IPC)方式,它使用了一个命名管道来实现客户端和服务器进程之间的通信。
以下是使用FIFO进行IPC的客户端/服务器应用程序的简单代码示例:
服务器代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/stat.h>
int main()
{
int fd, client_fd;
char *fifo = "server_fifo";
int number = 0;
int read_result;
char client_request[100];
// 创建FIFO文件
umask(0);
mknod(fifo, S_IFIFO | 0666, 0);
// 打开FIFO文件,并监听客户端请求
while (1)
{
fd = open(fifo, O_RDONLY);
read_result = read(fd, client_request, 100);
client_request[read_result] = '\0';
close(fd);
// 如果读到客户端请求,向客户端发送一个唯一的顺序数字
if (read_result > 0)
{
number++;
sprintf(client_request, "%d", number);
client_fd = open(client_request, O_WRONLY);
write(client_fd, &number, sizeof(number));
close(client_fd);
}
}
return 0;
}
```
客户端代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/stat.h>
int main()
{
int fd;
char *fifo = "server_fifo";
int number;
// 向服务器发送请求
fd = open(fifo, O_WRONLY);
write(fd, getprogname(), strlen(getprogname()) + 1);
close(fd);
// 获取服务器发送
相关推荐
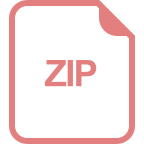
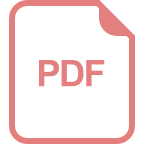
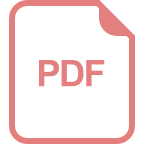














