如何在不使用Spring框架的情况下,在第三方代码中调用Feign客户端?
时间: 2024-11-30 11:18:56 浏览: 14
在不使用Spring框架的情况下直接调用Feign客户端,你可以按照以下步骤操作:
1. **添加依赖**:首先,你需要在项目中引入Feign的核心库和其他相关的依赖,例如Netflix Ribbon(用于负载均衡),以及Hystrix(断路器管理)。如果你的应用是一个Java应用,可以添加以下Maven或Gradle依赖:
```xml
<!-- Maven -->
<dependency>
<groupId>io.github.openfeign</groupId>
<artifactId>feign-core</artifactId>
<version>9.7.0</version> <!-- 更新至最新版本 -->
</dependency>
<dependency>
<groupId>com.netflix.ribbon</groupId>
<artifactId>ribbon</artifactId>
<version>2.2.24</version> <!-- 更新至最新版本 -->
</dependency>
<dependency>
<groupId>com.netflix.hystrix</groupId>
<artifactId>hystrix-core</artifactId>
<version>1.5.18</version> <!-- 更新至最新版本 -->
</dependency>
<!-- Gradle (如果使用的是Kotlin,可能会有额外的依赖处理,如ktor-client-serialization等) -->
implementation 'io.github.openfeign:feign-core:9.7.0'
implementation 'com.netflix.ribbon:ribbon:2.2.24'
implementation 'com.netflix.hystrix:hystrix-core:1.5.18'
```
2. **创建Feign接口**:为你需要访问的服务定义一个Feign接口,声明HTTP请求方法和返回类型。例如,对于一个REST API服务,可能像这样:
```java
public interface MyService {
@GetMapping("/api/v1/users")
User getUsers(@RequestParam("id") Long userId);
// 添加其他API方法...
}
```
3. **实现Feign Client**:创建一个实现了该接口的类,并提供实际的调用逻辑。这通常是通过`@FeignClient`注解指定远程服务URL,然后在对应方法上注入具体的客户端实现:
```java
import feign.Feign;
@FeignClient(name = "my-service", url = "http://example.com/api/v1")
public class FeignMyService implements MyService {
private final RestTemplate restTemplate; // 使用默认的HTTP客户端,如Retrofit或OkHttp
public FeignMyService() {
this.restTemplate = new RestTemplate();
}
@Override
public User getUsers(Long userId) {
return restTemplate.getForObject(String.format("/api/v1/users/%d", userId), User.class);
}
// 实现其他接口方法
}
```
4. **使用Feign Client**:现在你可以通过实例化这个FeignMyService类并调用其方法来执行远程调用了:
```java
public static void main(String[] args) {
FeignMyService service = new FeignMyService();
User user = service.getUsers(1L); // 调用API获取用户信息
System.out.println(user);
}
```
阅读全文
相关推荐
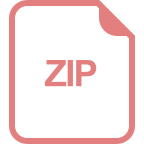
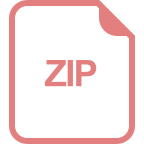
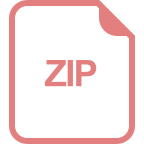

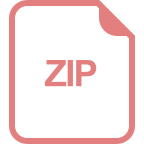
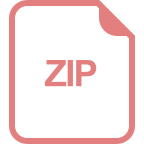
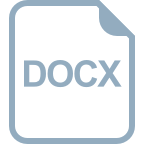
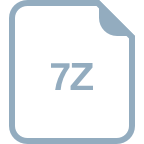
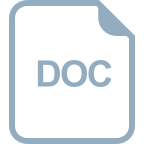
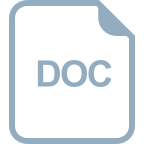
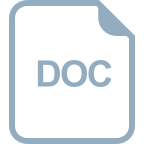
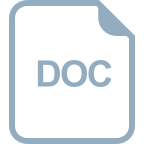
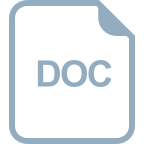
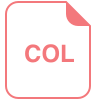
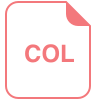
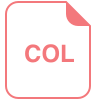
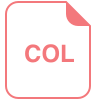
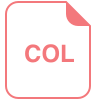
