self.driver.implicitly_wait(20)
时间: 2024-06-14 10:07:53 浏览: 15
`self.driver.implicitly_wait(20)`是Selenium中的一个方法,用于设置隐式等待时间。它的作用是在查找元素时,如果元素没有立即出现,会等待一定的时间再进行查找。这个方法的参数是等待的时间,单位是秒。
以下是一个演示示例:
```python
from selenium import webdriver
driver = webdriver.Chrome()
driver.implicitly_wait(20) # 设置隐式等待时间为20秒
driver.get("http://www.baidu.com")
driver.find_element_by_id("kw").send_keys("范冰冰")
```
在这个示例中,我们首先创建了一个Chrome浏览器的实例,然后使用`implicitly_wait`方法设置了隐式等待时间为20秒。接下来,我们打开百度首页,并在搜索框中输入了"范冰冰"。
注意:`implicitly_wait`方法只对后续的元素查找操作起作用,对于已经存在的元素不会进行等待。另外,如果在指定的时间内找到了元素,那么程序会立即执行后续的操作,不会等待整个时间。
相关问题
def test_10(self): self.driver.get("http://oa.hhero.com.cn/?m=login")#访问网址 self.driver.set_window_size(1051, 798)#设置窗口大小 self.driver.find_element(By.NAME, "adminuser").click()#点击用户名输入框 self.driver.find_element(By.NAME, "adminuser").send_keys("admin")#输入用户名 self.driver.find_element(By.CSS_SELECTOR, "div:nth-child(3) .input").click()#点击密码输入框 self.driver.find_element(By.CSS_SELECTOR, "div:nth-child(3) .input").send_keys("a123456")#输入密码 self.driver.find_element(By.NAME, "button").click()#点击登录按钮 self.driver.implicitly_wait(5)#隐式等待5s self.driver.find_element(By.ID, "indesearchmenu").click()#点击搜索 self.driver.find_element(By.ID, "confirm_input").click()#点击搜索跳出的输入框 self.driver.find_element(By.ID, "confirm_input").send_keys("流程")#输入关键词"流程" self.driver.find_element(By.ID, "confirm_btn1").click()#点击确定 self.driver.find_element(By.LINK_TEXT, "流程模块列表").click()#点击"流程模块列表" self.driver.find_element(By.XPATH, "/html/body/table/tbody/tr/td[3]/div/div[4]/div/div[2]/div[1]/table/tbody/tr/td[2]/div/input").click()#在流程模块列表界面点击输入框 self.driver.find_element(By.XPATH, "/html/body/table/tbody/tr/td[3]/div/div[4]/div/div[2]/div[1]/table/tbody/tr/td[2]/div/input").send_keys("图书管理")#在输入框中输入图书管理 self.driver.find_element(By.CSS_SELECTOR, ".input-group-btn > .btn").click()#点击搜索 self.vars["types"] = self.driver.find_element(By.XPATH, "/html/body/table/tbody/tr/td[3]/div/div[4]/div/div[2]/div[3]/div[1]/table/tbody/tr/td[2]").text#获取其类型 print("{}".format(self.vars["types"])) 这段代码的测试用例都有哪些
这段代码包含了一个名为 `test_10` 的测试用例。该测试用例包括以下步骤:
1. 使用 `get()` 方法访问网址 "http://oa.hhero.com.cn/?m=login"。
2. 使用 `set_window_size()` 方法设置浏览器窗口大小为 (1051, 798)。
3. 使用 `find_element()` 方法和 `click()` 方法分别点击用户名输入框和密码输入框。
4. 使用 `find_element()` 方法和 `send_keys()` 方法分别输入用户名 "admin" 和密码 "a123456"。
5. 使用 `find_element()` 方法和 `click()` 方法点击登录按钮。
6. 使用 `implicitly_wait()` 方法隐式等待 5 秒。
7. 使用 `find_element()` 方法和 `click()` 方法分别点击搜索和搜索跳出的输入框。
8. 使用 `find_element()` 方法和 `send_keys()` 方法输入关键词 "流程"。
9. 使用 `find_element()` 方法和 `click()` 方法点击确定按钮。
10. 使用 `find_element()` 方法和 `click()` 方法点击 "流程模块列表"。
11. 使用 `find_element()` 方法和 `click()` 方法在流程模块列表界面点击输入框。
12. 使用 `find_element()` 方法和 `send_keys()` 方法在输入框中输入 "图书管理"。
13. 使用 `find_element()` 方法和 `click()` 方法点击搜索按钮。
14. 使用 `find_element()` 方法获取元素的文本,并将其赋值给字典变量 `self.vars["types"]`。
15. 使用 `print()` 函数打印出字典变量 `self.vars["types"]` 的值。
这个测试用例的目的是在指定的网页中进行一系列的操作,并获取特定元素的文本值进行验证。这些步骤可以用于测试网页的搜索功能,并验证搜索结果中的类型。
import os import time import platform from selenium import webdriver as webdriver1 from selenium.webdriver.ie.options import Options from selenium.webdriver.support import expected_conditions as EC from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.common.by import By from config import global_wait,root_url,use_edge,iedriver_path,edgedriver_path,chromedriver_path from public.basiclogger import LOGGING class BaseCase(object): '''基础用例''' def get_web_driver(self,url,data=None,browser='ie'): if browser=='chrome': #if platform.system()=="Windows": # os.system(r'taskkill /f /im chrome.exe') #else: # os.system(r"ps aux |grep chrome|awk '{print $2}'|xargs -i kill {}") options = webdriver1.ChromeOptions() options.add_experimental_option('excludeSwitches', ['enable-logging']) self.driver = webdriver1.Chrome(executable_path=chromedriver_path,options=options) self.driver.get(url) self.driver.maximize_window() self.driver.implicitly_wait(global_wait) else: #启动IE之前先杀死电脑上所有的IE if use_edge: os.system(r'taskkill /f /im msedge.exe') else: os.system(r'taskkill /f /im iexplore.exe') if use_edge: file1=edgedriver_path else: file1=iedriver_path options = Options() options.ignore_protected_mode_settings = True options.ignore_zoom_level = True if use_edge: options.attach_to_edge_chrome = True options.edge_executable_path = r"C:\Program Files (x86)\Microsoft\Edge\Application\msedge.exe" else: options.add_argument('--user-agent="Mozilla/5.0 (Windows NT 10.0; WOW64; Trident/7.0; rv:11.0) like Gecko"') self.driver=webdriver1.Ie(executable_path=file1,options=options) self.driver.get(url) self.driver.maximize_window() self.driver.implicitly_wait(global_wait) def get_logger(self): #创建日志文件名 filename=self.data["case_name"]+'_'+time.strftime("%Y-%m-%d_%H-%M-%S") #保存连接 filename1=os.path.join(r'test_log',filename+r'.log') LOGGING1=LOGGING(log_name=filename,log_dir="test_log") self.logger=LOGGING1.logObject self.LOGGER=LOGGING1
在您提供的代码中,第一行需要将多个导入语句分开,即:
```
import os
import time
import platform
from selenium import webdriver as webdriver1
from selenium.webdriver.ie.options import Options
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.common.by import By
from config import global_wait,root_url,use_edge,iedriver_path,edgedriver_path,chromedriver_path
from public.basiclogger import LOGGING
```
此外,我还注意到您的代码缩进存在问题,建议仔细检查缩进是否正确,以确保代码可以正确执行。
相关推荐
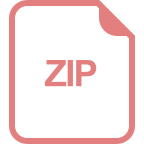
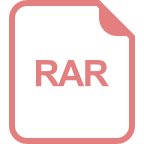
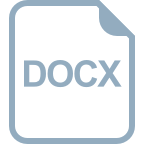












