如何使用C语言来解决上述问题?
时间: 2024-12-16 17:16:53 浏览: 4
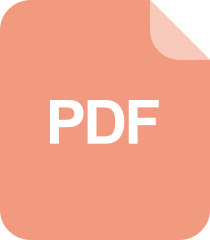
c语言来实现贪心算法之装箱问题
在C语言中解决计算三个点围成三角形周长的问题,可以采用相似的方式来处理。由于C语言没有内置的结构体和函数库支持复杂数学运算,我们需要手动编写一些函数来完成这个任务。
1. 首先,我们可以定义一个结构体`coord`来存储坐标信息:
```c
typedef struct {
double x;
double y;
} coord;
```
2. 创建函数计算两点之间的距离,这里假设给定两个点`a`和`b`:
```c
double distance(coord a, coord b) {
double dx = a.x - b.x;
double dy = a.y - b.y;
return sqrt(dx * dx + dy * dy); // 使用math.h头文件中的sqrt
}
```
注意:C标准库`math.h`包含了`sqrt`函数,但在许多系统上可能需要链接`mathlib`或`m`库。
3. 然后定义一个函数用于计算三角形的周长:
```c
double triangle_perimeter(coord p1, coord p2, coord p3) {
return distance(p1, p2) + distance(p2, p3) + distance(p3, p1);
}
```
4. 主函数接收用户输入,并调用上面定义的函数:
```c
#include <stdio.h>
#include <stdlib.h> // 包含sqrt函数
#include <math.h>
int main() {
coord p1, p2, p3;
printf("请输入第一个点的坐标 (x, y): ");
scanf("%lf %lf", &p1.x, &p1.y);
printf("请输入第二个点的坐标: ");
scanf("%lf %lf", &p2.x, &p2.y);
printf("请输入第三个点的坐标: ");
scanf("%lf %lf", &p3.x, &p3.y);
double perimeter = triangle_perimeter(p1, p2, p3);
printf("三个点围成的三角形周长是: %.2lf\n", perimeter); // 控制精度到两位小数
return 0;
}
```
阅读全文
相关推荐
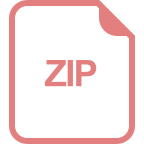
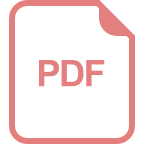
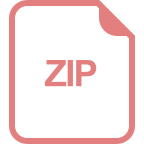
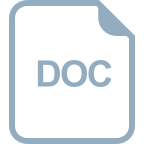
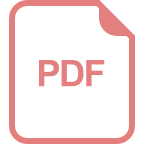
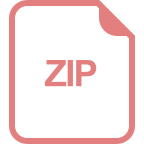
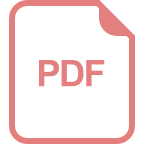
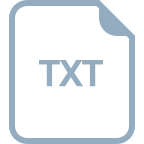
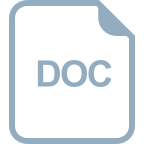
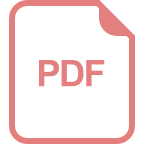
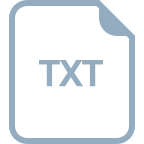
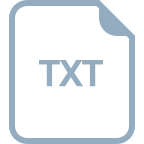
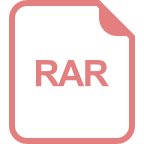
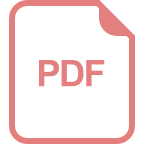
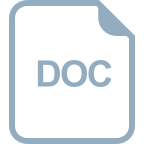
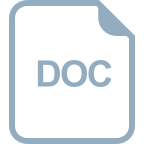
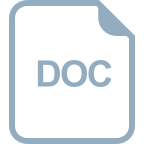