what is the unwrap2D and ftp functions
时间: 2024-03-07 14:49:40 浏览: 65
`unwrap2D` and `ftp` are custom functions that need to be implemented separately in MATLAB.
`unwrap2D` is a function that performs 2D phase unwrapping. It takes in two phase maps (`phase_map1` and `phase_map2`) and combines them to get the unwrapped phase map. This function is commonly used in FTP to avoid phase wrapping errors.
Here is an example implementation of `unwrap2D` function:
```matlab
function phase_unwrapped = unwrap2D(phase_map1, phase_map2)
% Calculate the 2D phase difference
phase_diff = phase_map1 - phase_map2;
% Set the center pixel to zero to avoid phase jumps
phase_diff(floor(size(phase_diff,1)/2)+1, floor(size(phase_diff,2)/2)+1) = 0;
% Perform 2D phase unwrapping
phase_unwrapped = unwrap(angle(exp(1i*phase_diff)));
% Set the center pixel back to zero
phase_unwrapped(floor(size(phase_unwrapped,1)/2)+1, floor(size(phase_unwrapped,2)/2)+1) = 0;
end
```
`ftp` is a function that performs Fourier transform profilometry on the unwrapped phase map to obtain the height map. It applies a 2D Fourier transform on the phase map and uses the frequency domain information to extract the height information.
Here is an example implementation of the `ftp` function:
```matlab
function height_map = ftp(phase_unwrapped)
% Calculate the Fourier spectrum of the phase map
F = fft2(phase_unwrapped);
% Shift the Fourier spectrum to center it
F = fftshift(F);
% Calculate the frequency domain coordinates
[X,Y] = meshgrid(1:size(F,2), 1:size(F,1));
x = X - size(F,2)/2 - 1;
y = Y - size(F,1)/2 - 1;
% Calculate the height map using the inverse Fourier transform
height_map = ifft2(1i*2*pi*(x.*F + y.*F))./abs(F).^2;
% Shift the height map to center it
height_map = fftshift(height_map);
end
```
Note: These are just example implementations of `unwrap2D` and `ftp` functions and may not be optimized for speed or accuracy.
阅读全文
相关推荐
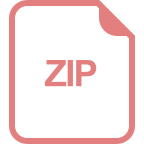
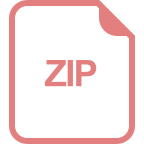
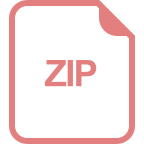
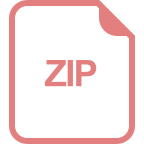
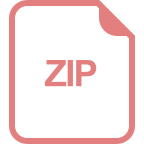
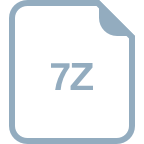
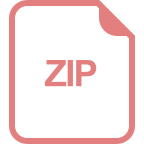
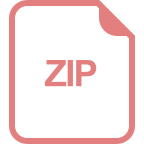







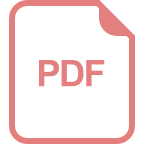
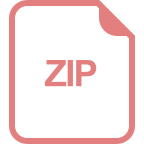