用c语言编写一个主函数,调试上述算法。随机产生或键盘输入一组元素,建立一个带头结点的单向链表 遍历单向链表。 在单向链表中删除所有的偶数元素结点。 编写在非递减有序链表中插入一个元素使链表元素仍有序的函数,并利 用该函数建立一个非递减有序单向链表。
时间: 2024-11-24 17:40:20 浏览: 32
在C语言中,首先我们需要定义链表结构,包含节点的数据域和指向下一个节点的指针。接下来,我们可以按照以下步骤编写相应的函数:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h> // 需要用到时间库生成随机数
// 定义链表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 初始化链表头节点
Node* createList() {
Node* head = (Node*)malloc(sizeof(Node));
if (!head) {
printf("Memory allocation failed.\n");
return NULL;
}
head->data = 0; // 或者随机生成第一个元素
head->next = NULL;
return head;
}
// 打印链表
void printList(Node* head) {
Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
// 删除偶数节点
Node* deleteEvenNodes(Node* head) {
Node* current = head;
Node* prev = NULL;
while (current != NULL) {
if (current->data % 2 == 0) {
if (prev != NULL) {
prev->next = current->next;
} else {
head = current->next;
}
free(current); // 释放内存
current = current->next;
} else {
prev = current;
current = current->next;
}
}
return head;
}
// 插入保持非递减顺序的函数
Node* insertSorted(Node* head, int value) {
if (head == NULL || value <= head->data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = head;
head = newNode;
return head;
}
Node* current = head;
while (current->next != NULL && current->next->data > value) {
current = current->next;
}
if (current->next != NULL) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = current->next;
current->next = newNode;
} else {
newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
current->next = newNode;
}
return head;
}
int main() {
srand(time(0)); // 设置随机数种子
Node* list = createList();
// 随机生成或输入元素(这里仅示例随机生成)
for (int i = 0; i < 10; ++i) {
list = insertSorted(list, rand() % 50); // 随机值在0-49之间
}
printList(list);
list = deleteEvenNodes(list);
printList(list);
free(head); // 清理不再需要的链表
return 0;
}
```
阅读全文
相关推荐




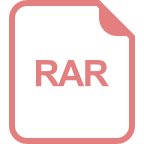













