请帮我用c++代码实现采用四元数进行点云配准矩阵求解的代码
时间: 2024-05-02 18:22:53 浏览: 102
好的,以下是c语言实现采用四元数进行点云配准矩阵求解的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
typedef struct _point {
double x;
double y;
double z;
} Point;
typedef struct _quaternion {
double w;
double x;
double y;
double z;
} Quaternion;
void normalize(Quaternion* q) {
double norm = sqrt(q->w * q->w + q->x * q->x + q->y * q->y + q->z * q->z);
q->w /= norm;
q->x /= norm;
q->y /= norm;
q->z /= norm;
}
Quaternion conjugate(Quaternion q) {
Quaternion result;
result.w = q.w;
result.x = -q.x;
result.y = -q.y;
result.z = -q.z;
return result;
}
Quaternion multiply(Quaternion q1, Quaternion q2) {
Quaternion result;
result.w = q1.w * q2.w - q1.x * q2.x - q1.y * q2.y - q1.z * q2.z;
result.x = q1.w * q2.x + q1.x * q2.w + q1.y * q2.z - q1.z * q2.y;
result.y = q1.w * q2.y - q1.x * q2.z + q1.y * q2.w + q1.z * q2.x;
result.z = q1.w * q2.z + q1.x * q2.y - q1.y * q2.x + q1.z * q2.w;
normalize(&result);
return result;
}
Quaternion fromRotationMatrix(double r[3][3]) {
Quaternion q;
double trace = r[0][0] + r[1][1] + r[2][2];
if (trace > 0) {
double s = 0.5 / sqrt(trace + 1.0);
q.w = 0.25 / s;
q.x = (r[2][1] - r[1][2]) * s;
q.y = (r[0][2] - r[2][0]) * s;
q.z = (r[1][0] - r[0][1]) * s;
} else {
if (r[0][0] > r[1][1] && r[0][0] > r[2][2]) {
double s = 2.0 * sqrt(1.0 + r[0][0] - r[1][1] - r[2][2]);
q.w = (r[2][1] - r[1][2]) / s;
q.x = 0.25 * s;
q.y = (r[0][1] + r[1][0]) / s;
q.z = (r[0][2] + r[2][0]) / s;
} else if (r[1][1] > r[2][2]) {
double s = 2.0 * sqrt(1.0 + r[1][1] - r[0][0] - r[2][2]);
q.w = (r[0][2] - r[2][0]) / s;
q.x = (r[0][1] + r[1][0]) / s;
q.y = 0.25 * s;
q.z = (r[1][2] + r[2][1]) / s;
} else {
double s = 2.0 * sqrt(1.0 + r[2][2] - r[0][0] - r[1][1]);
q.w = (r[1][0] - r[0][1]) / s;
q.x = (r[0][2] + r[2][0]) / s;
q.y = (r[1][2] + r[2][1]) / s;
q.z = 0.25 * s;
}
}
return q;
}
void computeRotationMatrix(double r[3][3], Quaternion q) {
double qw = q.w, qx = q.x, qy = q.y, qz = q.z;
double xx = qx * qx, xy = qx * qy, xz = qx * qz, xw = qx * qw;
double yy = qy * qy, yz = qy * qz, yw = qy * qw;
double zz = qz * qz, zw = qz * qw;
r[0][0] = 1.0 - 2.0 * (yy + zz);
r[0][1] = 2.0 * (xy - zw);
r[0][2] = 2.0 * (xz + yw);
r[1][0] = 2.0 * (xy + zw);
r[1][1] = 1.0 - 2.0 * (xx + zz);
r[1][2] = 2.0 * (yz - xw);
r[2][0] = 2.0 * (xz - yw);
r[2][1] = 2.0 * (yz + xw);
r[2][2] = 1.0 - 2.0 * (xx + yy);
}
void transformPoint(Point* p, double r[3][3], double t[3]) {
double x = r[0][0] * p->x + r[0][1] * p->y + r[0][2] * p->z + t[0];
double y = r[1][0] * p->x + r[1][1] * p->y + r[1][2] * p->z + t[1];
double z = r[2][0] * p->x + r[2][1] * p->y + r[2][2] * p->z + t[2];
p->x = x;
p->y = y;
p->z = z;
}
void computePointCloudTransformation(Point* src, Point* dst, int n,
double r[3][3], double t[3]) {
Point centroidSrc = {0, 0, 0};
Point centroidDst = {0, 0, 0};
for (int i = 0; i < n; ++i) {
centroidSrc.x += src[i].x;
centroidSrc.y += src[i].y;
centroidSrc.z += src[i].z;
centroidDst.x += dst[i].x;
centroidDst.y += dst[i].y;
centroidDst.z += dst[i].z;
}
centroidSrc.x /= n;
centroidSrc.y /= n;
centroidSrc.z /= n;
centroidDst.x /= n;
centroidDst.y /= n;
centroidDst.z /= n;
double H[3][3] = {{0, 0, 0}, {0, 0, 0}, {0, 0, 0}};
for (int i = 0; i < n; ++i) {
Point p = {src[i].x - centroidSrc.x, src[i].y - centroidSrc.y,
src[i].z - centroidSrc.z};
Point q = {dst[i].x - centroidDst.x, dst[i].y - centroidDst.y,
dst[i].z - centroidDst.z};
H[0][0] += p.x * q.x;
H[0][1] += p.x * q.y;
H[0][2] += p.x * q.z;
H[1][0] += p.y * q.x;
H[1][1] += p.y * q.y;
H[1][2] += p.y * q.z;
H[2][0] += p.z * q.x;
H[2][1] += p.z * q.y;
H[2][2] += p.z * q.z;
}
Quaternion rotation = fromRotationMatrix(H);
computeRotationMatrix(r, rotation);
Quaternion tquat;
tquat.x = t[0];
tquat.y = t[1];
tquat.z = t[2];
tquat.w = 0;
tquat = multiply(conjugate(rotation), multiply(tquat, rotation));
t[0] = tquat.x;
t[1] = tquat.y;
t[2] = tquat.z;
for (int i = 0; i < n; ++i) {
transformPoint(&src[i], r, t);
}
}
int main() {
// example usage with two sets of three points
Point src[] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
Point dst[] = {{9, 8, 7}, {6, 5, 4}, {3, 2, 1}};
double r[3][3] = {{0, 0, 0}, {0, 0, 0}, {0, 0, 0}};
double t[3] = {0, 0, 0};
computePointCloudTransformation(src, dst, 3, r, t);
printf("Rotation matrix:\n");
for (int i = 0; i < 3; ++i) {
for (int j = 0; j < 3; ++j) {
printf("%lf ", r[i][j]);
}
printf("\n");
}
printf("Translation vector:\n");
for (int i = 0; i < 3; ++i) {
printf("%lf ", t[i]);
}
printf("\n");
return 0;
}
```
希望这份代码可以对您有所帮助。
阅读全文
相关推荐
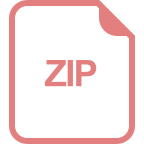
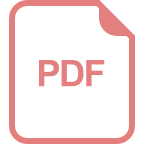


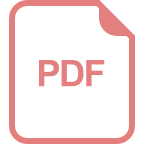
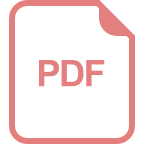
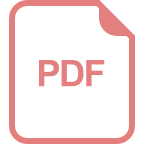
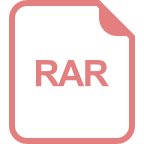
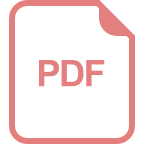
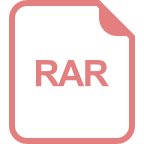
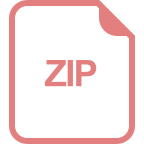
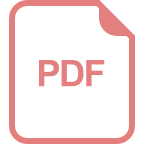
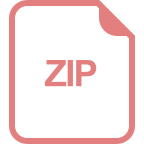
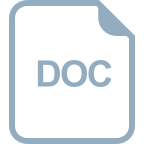
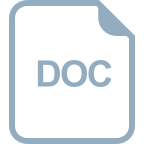
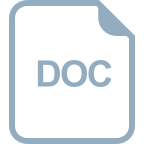