用python写一个获取进程句柄的函数
时间: 2024-02-06 17:12:21 浏览: 28
好的,以下是一个获取指定进程名的句柄的Python函数:
``` python
import win32com.client
def get_process_handle(process_name):
"""
获取指定进程名的句柄
:param process_name: 进程名
:return: 进程句柄
"""
wmi = win32com.client.GetObject('winmgmts:')
processes = wmi.InstancesOf('win32_process')
for process in processes:
if process.Name == process_name:
return process.Handle
```
该函数使用了 `win32com` 库来获取当前系统中所有进程的信息,并遍历这些进程,查找与指定进程名相匹配的进程,并返回其句柄。需要注意的是,该函数只返回第一个匹配的进程句柄,如果需要获取多个进程的句柄,需要在函数内部进行修改。
相关问题
用Python写一个获取app句柄的代码
可以使用Python中的`win32gui`模块来获取app的句柄。以下是一个获取指定窗口句柄的示例代码:
```python
import win32gui
# 定义一个回调函数,用于找到指定类名和窗口名的窗口
def find_window_handler(hwnd, window_name):
if win32gui.IsWindowVisible(hwnd) and window_name in win32gui.GetWindowText(hwnd):
print("找到窗口:{}".format(win32gui.GetWindowText(hwnd)))
return hwnd
# 查找窗口句柄
hwnd = win32gui.EnumWindows(find_window_handler, "窗口标题")
```
上述代码中,`find_window_handler`是一个回调函数,用于判断当前枚举到的窗口是否为指定窗口。`EnumWindows`函数会遍历当前系统中所有的顶级窗口,并对每个窗口调用`find_window_handler`函数。如果找到了指定窗口,则返回窗口句柄。
在上述代码中,我们将窗口标题作为参数传递给`find_window_handler`函数。如果你要获取的是其他信息,如窗口类名、进程ID等,可以在`find_window_handler`函数中使用`win32gui`模块提供的相应API函数来获取。
Python 获取进程中的句柄
在 Python 中可以使用 `ctypes` 库来获取进程中的句柄。您可以使用 `ctypes.windll.kernel32` 模块调用 Windows API 函数 `OpenProcess` 来打开一个进程,并获取该进程的句柄。示例代码如下:
```
import ctypes
import sys
PROCESS_QUERY_INFORMATION = 0x0400
PROCESS_VM_READ = 0x0010
def get_process_handle(pid):
handle = ctypes.windll.kernel32.OpenProcess(PROCESS_QUERY_INFORMATION | PROCESS_VM_READ, False, pid)
if handle == 0:
sys.exit("Could not acquire process handle")
return handle
pid = 1234 # Replace with the PID of the process you want to get a handle for
handle = get_process_handle(pid)
print("Handle:", handle)
```
在这个例子中,您需要替换 `pid` 变量为您要获取句柄的进程的 PID。
相关推荐
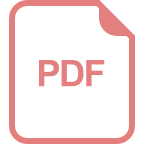












