粒子群算法python路径规划每个粒子两伟编码并赋初值
时间: 2023-09-09 13:13:12 浏览: 83
以下是一个简单的粒子群算法的python实现,用于路径规划。每个粒子的位置由两个维度的编码表示,并赋予初始值。在此示例中,我们将使用一个二维平面,其中每个粒子的位置由x和y坐标组成。
```python
import random
# 定义粒子类
class Particle:
def __init__(self, x_min, x_max, y_min, y_max):
self.x = random.uniform(x_min, x_max)
self.y = random.uniform(y_min, y_max)
self.velocity_x = 0
self.velocity_y = 0
self.best_x = self.x
self.best_y = self.y
self.fitness = float('inf')
# 更新粒子位置和速度
def update(self, w, c1, c2, global_best_x, global_best_y):
r1 = random.uniform(0, 1)
r2 = random.uniform(0, 1)
self.velocity_x = w * self.velocity_x + c1 * r1 * (self.best_x - self.x) + c2 * r2 * (global_best_x - self.x)
self.velocity_y = w * self.velocity_y + c1 * r1 * (self.best_y - self.y) + c2 * r2 * (global_best_y - self.y)
self.x += self.velocity_x
self.y += self.velocity_y
# 更新最佳位置
if self.fitness < self.get_fitness(self.best_x, self.best_y):
self.best_x = self.x
self.best_y = self.y
# 计算适应度函数
def get_fitness(self, x, y):
# TODO: 根据实际问题定义适应度函数
return 0
# 初始化粒子群
def initialize_particles(num_particles, x_min, x_max, y_min, y_max):
particles = []
for i in range(num_particles):
particle = Particle(x_min, x_max, y_min, y_max)
particles.append(particle)
return particles
# 执行粒子群算法
def particle_swarm_optimization(num_iterations, num_particles, x_min, x_max, y_min, y_max, w, c1, c2):
particles = initialize_particles(num_particles, x_min, x_max, y_min, y_max)
global_best_x = float('inf')
global_best_y = float('inf')
for i in range(num_iterations):
for particle in particles:
fitness = particle.get_fitness(particle.x, particle.y)
if fitness < particle.fitness:
particle.fitness = fitness
particle.best_x = particle.x
particle.best_y = particle.y
if fitness < particle.get_fitness(global_best_x, global_best_y):
global_best_x = particle.x
global_best_y = particle.y
for particle in particles:
particle.update(w, c1, c2, global_best_x, global_best_y)
return global_best_x, global_best_y
```
在上面的代码中,我们定义了一个Particle类来表示粒子,每个粒子具有x和y坐标、速度和最佳位置。我们还定义了一个initialize_particles函数来初始化粒子群,以及一个particle_swarm_optimization函数来执行粒子群算法。
请注意,我们还定义了一个get_fitness函数来计算适应度函数。这个函数是根据实际问题定义的,它应该考虑到路径规划的目标和约束条件。
要使用这个例子,你需要将x_min、x_max、y_min和y_max设置为路径规划问题的范围,并将w、c1和c2设置为算法参数。你还需要定义一个适应度函数来计算每个粒子在给定位置的适应度。
例如,如果我们希望在一个10x10的网格中寻找从起点到终点的最短路径,我们可以将x_min、x_max、y_min和y_max设置为0和10,将w、c1和c2设置为常数,然后在get_fitness函数中使用一个常规的图搜索算法来计算路径长度。
阅读全文
相关推荐
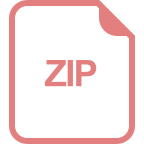
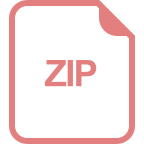
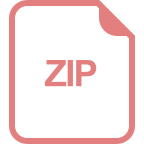
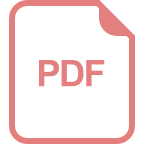
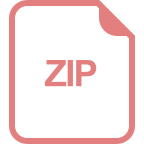
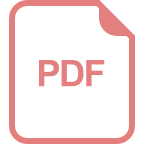
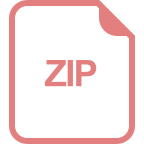
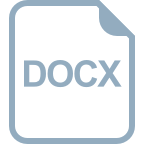
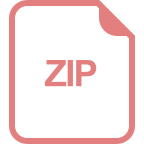
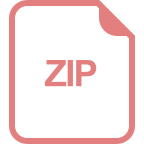
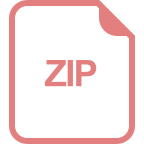
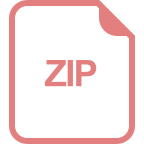
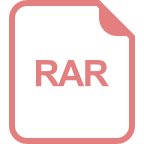