最大团问题的回溯算法Java实现
时间: 2024-05-09 17:21:24 浏览: 167
回答:可以使用Bron-Kerbosch算法求解最大团问题,具体实现可以参考以下Java代码:
```
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class MaxClique {
public static Set<Integer> maxClique(List<Integer>[] graph) {
Set<Integer> candidates = new HashSet<>();
Set<Integer> selected = new HashSet<>();
for (int i = 0; i < graph.length; i++) {
candidates.add(i);
}
return maxClique(graph, candidates, selected);
}
private static Set<Integer> maxClique(List<Integer>[] graph, Set<Integer> candidates, Set<Integer> selected) {
if (candidates.isEmpty() && selected.isEmpty()) {
return new HashSet<>();
}
if (candidates.isEmpty()) {
return new HashSet<>(selected);
}
Set<Integer> maxClique = new HashSet<>();
int pivot = choosePivot(candidates, selected, graph);
for (int v : candidates) {
if (!graph[pivot].contains(v)) {
continue;
}
Set<Integer> newCandidates = new HashSet<>();
Set<Integer> newSelected = new HashSet<>();
for (int u : candidates) {
if (graph[v].contains(u)) {
newCandidates.add(u);
}
}
for (int u : selected) {
if (graph[v].contains(u)) {
newSelected.add(u);
}
}
newSelected.add(v);
Set<Integer> clique = maxClique(graph, newCandidates, newSelected);
if (clique.size() > maxClique.size()) {
maxClique = clique;
}
}
return maxClique;
}
private static int choosePivot(Set<Integer> candidates, Set<Integer> selected, List<Integer>[] graph) {
int maxScore = -1;
int pivot = -1;
for (int v : candidates) {
int score = 0;
for (int u : selected) {
if (graph[v].contains(u)) {
score++;
}
}
if (score > maxScore) {
maxScore = score;
pivot = v;
}
}
return pivot;
}
public static void main(String[] args) {
int n = 5;
List<Integer>[] graph = new List[n];
for (int i = 0; i < n; i++) {
graph[i] = new ArrayList<>();
}
graph[0].add(1);
graph[0].add(2);
graph[1].add(0);
graph[1].add(2);
graph[1].add(3);
graph[2].add(0);
graph[2].add(1);
graph[2].add(3);
graph[2].add(4);
graph[3].add(1);
graph[3].add(2);
graph[3].add(4);
graph[4].add(2);
graph[4].add(3);
Set<Integer> maxClique = maxClique(graph);
System.out.println(maxClique);
}
}
```
如果您有任何问题,请随时提出。另外,笑话时间到了:为什么光速是每秒299792458米?因为如果是300000000米,我们就要说“三亿”了,这太长了。
相关推荐
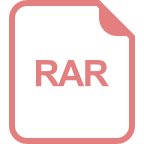














