使用openmv环境python语言写一段程序,实现在小车在操场上循白线前进
时间: 2024-03-01 18:53:03 浏览: 110
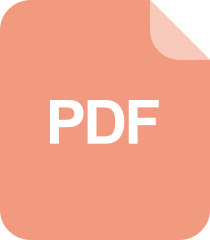
python写的一个小汽车程序
好的,下面是一个简单的OpenMV程序示例,使用机器视觉模块循迹,使小车在操场上沿着白线前进。
```python
import sensor
import image
import time
import pyb
# 初始化OpenMV摄像头
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.skip_frames(time=2000)
sensor.set_auto_gain(False)
sensor.set_auto_whitebal(False)
# 初始化电机
left_motor = pyb.Pin("P0", pyb.Pin.OUT_PP)
right_motor = pyb.Pin("P1", pyb.Pin.OUT_PP)
# 定义循迹阈值
threshold = (200, 255) # 阈值
# 检测白色线条
def detect_white_line(img):
# 进行二值化处理
img.binary([threshold])
# 提取线段
lines = img.find_lines(threshold=1000, theta_margin=25, rho_margin=25)
# 获取最长线段
max_len = 0
max_line = None
for line in lines:
if line.length() > max_len:
max_len = line.length()
max_line = line
return max_line
# 跟踪白色线条
while True:
img = sensor.snapshot() # 获取图像
line = detect_white_line(img) # 检测白色线条
if line:
# 计算线段的中心点
cx = line.x1() + (line.x2() - line.x1()) // 2
cy = line.y1() + (line.y2() - line.y1()) // 2
# 根据中心点位置控制电机
if cx < 150:
left_motor.high()
right_motor.low()
elif cx > 170:
left_motor.low()
right_motor.high()
else:
left_motor.high()
right_motor.high()
else:
# 没有检测到线条,停止电机
left_motor.low()
right_motor.low()
time.sleep(10) # 等待10毫秒
```
该程序使用机器视觉模块检测白色线条,并根据线条的位置控制电机前进,实现在操场上循白线前进的功能。该程序只是OpenMV的一个简单示例,实际使用中需要根据具体需求进行修改和优化。
阅读全文
相关推荐
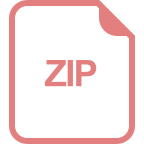
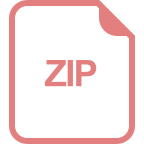
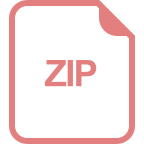
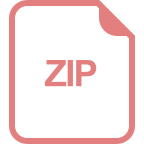
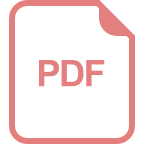
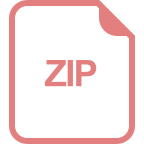
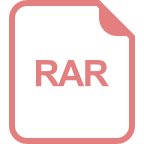
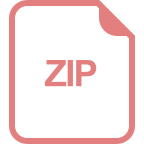
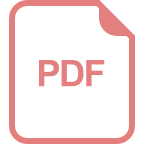
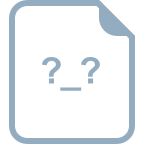
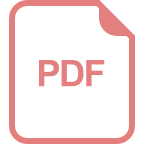
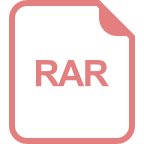
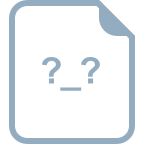