定义一个职工类Employee 数据成员 (private): 职工姓名 (char *name)、性别 (bool sex)、年龄、工龄、工资 成员函数 (public): 构造函数:职工基本信息的初始化(姓名、性别、年龄、工龄、工资) 析构函数:释放系统资源(堆空间) 修改职工姓名 修改工资 年薪计算(12×工资+年终奖) 工龄增加 显示职工信息用c++
时间: 2023-12-19 07:25:28 浏览: 135
以下是一个简单的Employee类的定义,包含上述要求的数据成员和成员函数:
```c++
class Employee {
public:
// 构造函数
Employee(const char* name, bool sex, int age, int seniority, double salary);
// 析构函数
~Employee();
// 修改职工姓名
void setName(const char* name);
// 修改工资
void setSalary(double salary);
// 年薪计算
double calcAnnualSalary() const;
// 工龄增加
void increaseSeniority(int years);
// 显示职工信息
void display() const;
private:
char* name; // 职工姓名
bool sex; // 性别
int age; // 年龄
int seniority; // 工龄
double salary; // 工资
};
```
下面是类成员函数的实现:
```c++
Employee::Employee(const char* name, bool sex, int age, int seniority, double salary) {
// 分配堆空间并复制姓名
this->name = new char[strlen(name) + 1];
strcpy(this->name, name);
// 初始化其他数据成员
this->sex = sex;
this->age = age;
this->seniority = seniority;
this->salary = salary;
}
Employee::~Employee() {
// 释放堆空间
delete[] name;
}
void Employee::setName(const char* name) {
// 释放原有的堆空间
delete[] this->name;
// 分配新的堆空间并复制姓名
this->name = new char[strlen(name) + 1];
strcpy(this->name, name);
}
void Employee::setSalary(double salary) {
this->salary = salary;
}
double Employee::calcAnnualSalary() const {
return 12 * salary + 10000; // 假设年终奖为10000元
}
void Employee::increaseSeniority(int years) {
seniority += years;
}
void Employee::display() const {
std::cout << "姓名:" << name << std::endl;
std::cout << "性别:" << (sex ? "男" : "女") << std::endl;
std::cout << "年龄:" << age << "岁" << std::endl;
std::cout << "工龄:" << seniority << "年" << std::endl;
std::cout << "工资:" << salary << "元/月" << std::endl;
}
```
使用时,可以先创建一个Employee对象,然后调用其各个成员函数来修改或获取对象的属性:
```c++
int main() {
Employee emp("张三", true, 30, 5, 8000);
emp.display();
emp.setName("李四");
emp.setSalary(9000);
emp.increaseSeniority(1);
std::cout << "年薪:" << emp.calcAnnualSalary() << "元" << std::endl;
emp.display();
return 0;
}
```
阅读全文
相关推荐
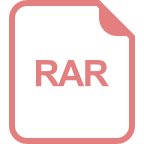




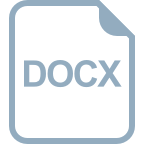
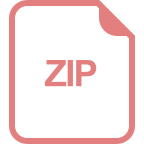
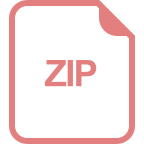