提供一个完整详细的基于图片的安全帽佩戴识别代码示例,要求只使用sklearn,机器学习
时间: 2024-11-17 07:29:39 浏览: 33
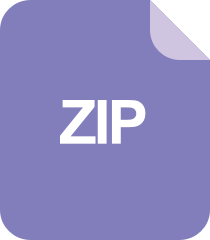
<项目代码>YOLOv8 安全帽佩戴识别<目标检测>
基于图片的安全帽佩戴识别通常涉及图像处理和机器学习模型。由于 `scikit-learn` 主要用于传统的机器学习算法,而不是深度学习,因此我们需要先对图像进行预处理,提取有用的特征,然后使用这些特征来训练模型。以下是一个完整的示例代码,展示了如何使用 `scikit-learn` 实现基于图片的安全帽佩戴识别:
### 1. 导入必要的库
```python
import os
import cv2
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score, classification_report
```
### 2. 图像预处理
```python
def preprocess_image(image_path):
# 读取图像
image = cv2.imread(image_path)
# 转换为灰度图
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 缩放图像到固定大小
resized = cv2.resize(gray, (64, 64))
# 将图像展平成一维数组
flattened = resized.flatten()
return flattened
def load_dataset(data_dir):
images = []
labels = []
for label in ['with_helmet', 'without_helmet']:
label_dir = os.path.join(data_dir, label)
for image_name in os.listdir(label_dir):
image_path = os.path.join(label_dir, image_name)
features = preprocess_image(image_path)
images.append(features)
labels.append(1 if label == 'with_helmet' else 0)
return np.array(images), np.array(labels)
```
### 3. 加载数据集
```python
data_dir = 'path_to_your_dataset'
X, y = load_dataset(data_dir)
```
### 4. 划分训练集和测试集
```python
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
### 5. 特征标准化
```python
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
```
### 6. 训练支持向量机(SVM)模型
```python
model = SVC(kernel='linear')
model.fit(X_train, y_train)
```
### 7. 预测和评估
```python
y_pred = model.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy:.2f}")
print("Classification Report:")
print(classification_report(y_test, y_pred))
```
### 8. 可视化结果(可选)
```python
import matplotlib.pyplot as plt
def plot_results(images, true_labels, predicted_labels, num_samples=10):
fig, axes = plt.subplots(2, num_samples // 2, figsize=(15, 6))
for i in range(num_samples):
ax = axes[i % 2, i // 2]
ax.imshow(images[i].reshape(64, 64), cmap='gray')
ax.set_title(f'True: {true_labels[i]}, Pred: {predicted_labels[i]}')
ax.axis('off')
plt.show()
plot_results(X_test[:10], y_test[:10], y_pred[:10])
```
### 9. 保存模型(可选)
```python
import joblib
joblib.dump(model, 'safety_helmet_model.pkl')
```
### 10. 加载模型并进行预测(可选)
```python
loaded_model = joblib.load('safety_helmet_model.pkl')
new_image_path = 'path_to_new_image.jpg'
new_features = preprocess_image(new_image_path).reshape(1, -1)
new_features = scaler.transform(new_features)
prediction = loaded_model.predict(new_features)
print(f"Prediction: {'With helmet' if prediction[0] == 1 else 'Without helmet'}")
```
这个示例代码展示了如何使用 `scikit-learn` 和传统机器学习方法(如支持向量机)进行基于图片的安全帽佩戴识别。请注意,对于更复杂的图像识别任务,建议使用深度学习框架(如 TensorFlow 或 PyTorch)。
阅读全文
相关推荐
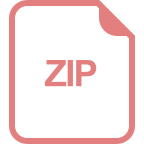
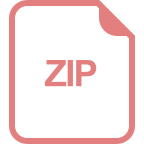
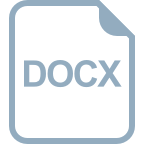
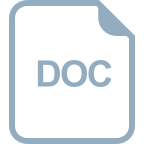
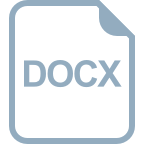
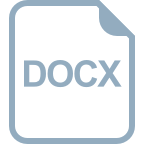
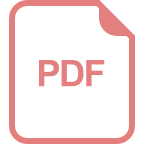
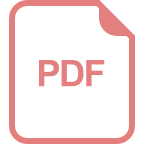
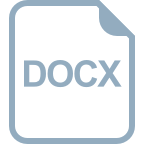
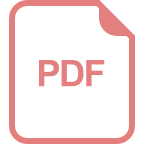
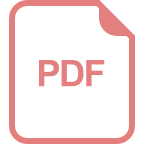
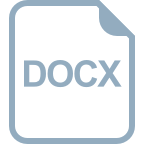
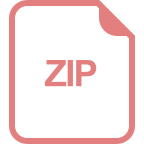
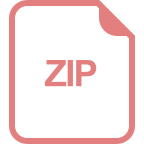
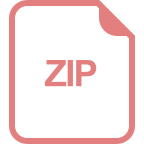
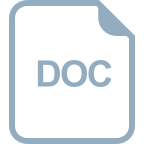
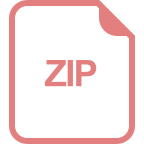