用python写的飞机大战的游戏内对敌人增加血条属性应该怎么做
时间: 2024-02-20 13:56:43 浏览: 178
要在飞机大战游戏中对敌人增加血条属性,你需要在游戏中为敌人对象添加一个属性来存储敌人的剩余血量,并在游戏界面上显示敌人的血条。
具体实现步骤如下:
1. 在敌人类中添加一个属性,用于存储敌人的剩余血量。例如:
```python
class Enemy(pygame.sprite.Sprite):
def __init__(self):
super().__init__()
self.image = pygame.image.load("enemy.png").convert_alpha()
self.rect = self.image.get_rect()
self.rect.x = random.randint(0, SCREEN_WIDTH - self.rect.width)
self.rect.y = -self.rect.height
self.speed = random.randint(1, 3)
self.health = 100 # 添加血量属性
```
2. 在游戏循环中更新敌人的血条。你可以在敌人对象中添加一个方法,用于返回敌人的血条信息。例如:
```python
class Enemy(pygame.sprite.Sprite):
def __init__(self):
...
self.health = 100
def get_health_bar(self):
# 计算血条长度
length = 50 * self.health / 100
# 创建一个 Surface 用于绘制血条
health_bar = pygame.Surface((50, 10))
# 根据血量设置血条颜色
if self.health > 75:
color = GREEN
elif self.health > 25:
color = YELLOW
else:
color = RED
# 绘制血条
pygame.draw.rect(health_bar, color, (0, 0, length, 10))
pygame.draw.rect(health_bar, WHITE, (0, 0, 50, 10), 2)
return health_bar
```
3. 在游戏循环中绘制敌人的血条。你可以在敌人对象的 `update()` 方法中调用 `get_health_bar()` 方法,然后将血条绘制到游戏界面上。例如:
```python
all_sprites.update()
...
for enemy in enemy_group:
enemy.update()
# 获取血条 Surface
health_bar = enemy.get_health_bar()
# 绘制血条
screen.blit(health_bar, (enemy.rect.x, enemy.rect.y - 10))
enemy_group.draw(screen)
```
这样,你就可以在飞机大战游戏中为敌人添加血条属性,并在游戏界面上显示敌人的血条了。
相关推荐
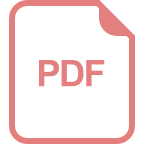












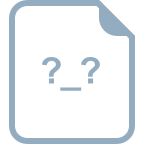