实验内容 1、创建数据库TEST,创建一个表student。 createdatabaseTEST; use TEST; create table student stu_name varchar(20), stu_sexVARCHAR(4), stu_age int, score int, PRIMARY KEY (stu name) );
时间: 2024-02-13 11:00:31 浏览: 105
好的,这是一个简单的SQL语句,用于创建一个名为TEST的数据库,其中包含一个名为student的表。表中包含stu_name、stu_sex、stu_age和score四个字段,并以stu_name字段作为主键。
```sql
CREATE DATABASE TEST;
USE TEST;
CREATE TABLE student (
stu_name VARCHAR(20),
stu_sex VARCHAR(4),
stu_age INT,
score INT,
PRIMARY KEY (stu_name)
);
```
执行以上SQL语句后,你就可以在MySQL中创建一个名为TEST的数据库,并在其中创建一个名为student的表了。如果你需要对这个表进行操作,可以使用类似以下的SQL语句:
```sql
-- 插入一条记录
INSERT INTO student (stu_name, stu_sex, stu_age, score) VALUES ('张三', '男', 20, 90);
-- 查询所有记录
SELECT * FROM student;
-- 更新一条记录
UPDATE student SET score = 95 WHERE stu_name = '张三';
-- 删除一条记录
DELETE FROM student WHERE stu_name = '张三';
```
希望这些示例能够帮助你更好地理解如何在MySQL中创建数据库和表,并进行基本的增删改查操作。
相关问题
1、创建数据库TEST,创建一个表student。 create database TEST; use TEST; create table student( stu_name varchar(20), stu_sex VARCHAR(4), stu_age int, score int, PRIMARY KEY (stu_name) ); 插入数据 insert into student(stu_name,stu_sex,stu_age,score) values('niuniu','男',15,90)2、定义数据库操作类JdbcAccess,定义三个方法: (1)getConnection():实现数据库的连接; (2)getStudent():指定学号,查询学生信息; (3)addStudent():将学生信息插入数据库。 3、定义主类,连接数据库、根据学号查询学生信息、插入学生信息等操作。;
好的,以下是对你的问题的回答:
首先,感谢您对ChitGPT的关注和使用。关于您的问题,我明白您需要创建一个名为TEST的数据库,并在其中创建一个名为student的表,然后插入一条学生记录。同时,您还需要定义一个名为JdbcAccess的数据库操作类,并在其中定义三个方法:getConnection()、getStudent()和addStudent()。最后,您需要创建一个主类,连接数据库并实现查询和插入学生信息等功能。
以下是相关的代码示例:
1、创建数据库TEST,创建一个表student:
```
create database TEST;
use TEST;
create table student(
stu_name varchar(20),
stu_sex VARCHAR(4),
stu_age int,
score int,
PRIMARY KEY (stu_name)
);
插入数据
insert into student(stu_name,stu_sex,stu_age,score) values('niuniu','男',15,90)
```
2、定义数据库操作类JdbcAccess,定义三个方法:
```
import java.sql.*;
public class JdbcAccess {
private static final String URL = "jdbc:mysql://localhost:3306/TEST?useSSL=false";
private static final String USER = "root";
private static final String PASSWORD = "123456";
// 获取数据库连接
public static Connection getConnection() throws SQLException {
return DriverManager.getConnection(URL, USER, PASSWORD);
}
// 根据学号获取学生信息
public static void getStudent(String stuName) throws SQLException {
Connection conn = getConnection();
String sql = "select * from student where stu_name=?";
PreparedStatement ps = conn.prepareStatement(sql);
ps.setString(1, stuName);
ResultSet rs = ps.executeQuery();
while (rs.next()) {
System.out.println("学生姓名:" + rs.getString("stu_name") + ",性别:" + rs.getString("stu_sex") + ",年龄:" + rs.getInt("stu_age") + ",分数:" + rs.getInt("score"));
}
rs.close();
ps.close();
conn.close();
}
// 插入学生信息
public static void addStudent(String stuName, String stuSex, int stuAge, int score) throws SQLException {
Connection conn = getConnection();
String sql = "insert into student(stu_name, stu_sex, stu_age, score) values(?, ?, ?, ?)";
PreparedStatement ps = conn.prepareStatement(sql);
ps.setString(1, stuName);
ps.setString(2, stuSex);
ps.setInt(3, stuAge);
ps.setInt(4, score);
ps.executeUpdate();
ps.close();
conn.close();
}
}
```
3、定义主类,连接数据库、根据学号查询学生信息、插入学生信息等操作。
```
import java.sql.SQLException;
public class Main {
public static void main(String[] args) {
try {
// 获取数据库连接
JdbcAccess.getConnection();
// 根据学号查询学生信息
JdbcAccess.getStudent("niuniu");
// 新增学生信息
JdbcAccess.addStudent("xiaoxue", "女", 16, 95);
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
以上是一个简单的示例,希望能对您有所帮助。如果您有任何问题或需要进一步的帮助,请随时联系我。
-- 如果存在则先删除 drop database if exists jzlg; -- 创建数据库 create database jzlg; -- 使用自己创建的数据库 use jzlg; -- 学生信息表 -- 如果存在则先删除 drop table if exists tb_student; -- 创建新表 create table tb_student( stu_id int primary key auto_increment, stu_name varchar(50) unique, create_date date, gender varchar(2), age int check(age>18 and age<24) )auto_increment=1; -- 查询 select * from tb_student; -- 学生成绩 -- 如果存在则先删除 drop table if exists tb_score; -- 创建新表 create table tb_score( score_id int primary key auto_increment, stu_id int, c decimal(4,2), s decimal(4,2), html decimal(4,2), constraint fk_stu_id foreign key (stu_id) references tb_student(stu_id) )auto_increment=1; -- 查询 select * from tb_score; 在这两张表里面添加数据每个表添加三条数据
以下是向两张表中添加三条数据的 SQL 语句:
向 tb_student 表中添加三条数据:
```
insert into tb_student(stu_name, create_date, gender, age) values('小明', '2002-01-01', '男', 19);
insert into tb_student(stu_name, create_date, gender, age) values('张三', '2003-02-02', '男', 20);
insert into tb_student(stu_name, create_date, gender, age) values('李四', '2004-03-03', '女', 21);
```
向 tb_score 表中添加三条数据:
```
insert into tb_score(stu_id, c, s, html) values(1, 80, 85, 90);
insert into tb_score(stu_id, c, s, html) values(2, 50, 40, 60);
insert into tb_score(stu_id, c, s, html) values(3, 45, 60, 25);
```
注意:在向 tb_score 表中插入数据时,需要保证 tb_student 表中已经存在对应的 stu_id。
阅读全文
相关推荐
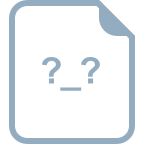
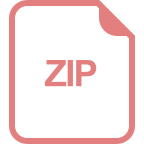
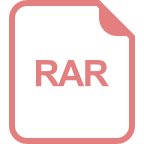
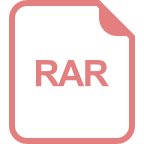








