linux怎么线程间通信
时间: 2024-12-08 21:11:25 浏览: 8
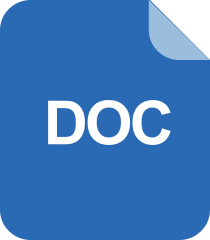
linux进程间通信 教程
在Linux系统中,线程间通信(Inter-Thread Communication, ITC)是一个重要的概念,主要用于在多线程环境中实现数据共享和协调。以下是几种常见的线程间通信方式:
1. **互斥锁(Mutex)**:
互斥锁用于保护共享资源,确保同一时间只有一个线程可以访问该资源。通过使用`pthread_mutex_lock`和`pthread_mutex_unlock`函数来实现锁的获取和释放。
2. **条件变量(Condition Variable)**:
条件变量用于线程间的同步,允许一个线程等待特定条件的发生。常用的函数有`pthread_cond_wait`和`pthread_cond_signal`,用于等待和唤醒线程。
3. **信号量(Semaphore)**:
信号量用于控制对共享资源的访问数量。它允许多个线程同时访问资源,但可以通过设置计数器来限制同时访问的线程数。常用的函数有`sem_wait`和`sem_post`。
4. **消息队列(Message Queue)**:
消息队列用于在不同线程之间传递消息。线程可以向队列中发送消息,也可以从队列中接收消息。Linux提供了POSIX消息队列和System V消息队列两种实现方式。
5. **共享内存(Shared Memory)**:
共享内存允许多个线程访问同一块内存区域,从而实现数据共享。这是最高效的IPC方式,但需要手动同步访问以避免数据竞争。
6. **管道(Pipes)和命名管道(FIFOs)**:
管道用于在父子进程之间或不同进程之间传递数据。命名管道(FIFOs)可以在不相关的进程之间传递数据。
### 示例代码:使用互斥锁和条件变量
```c
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
pthread_mutex_t mutex;
pthread_cond_t cond;
int count = 0;
void* increment(void* arg) {
for (int i = 0; i < 5; i++) {
pthread_mutex_lock(&mutex);
count++;
printf("Incremented count to %d\n", count);
pthread_cond_signal(&cond);
pthread_mutex_unlock(&mutex);
sleep(1);
}
return NULL;
}
void* watch(void* arg) {
while (count < 5) {
pthread_mutex_lock(&mutex);
while (count < 5) {
pthread_cond_wait(&cond, &mutex);
}
printf("Count is now %d\n", count);
pthread_mutex_unlock(&mutex);
}
return NULL;
}
int main() {
pthread_t inc_thread, watch_thread;
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&cond, NULL);
pthread_create(&inc_thread, NULL, increment, NULL);
pthread_create(&watch_thread, NULL, watch, NULL);
pthread_join(inc_thread, NULL);
pthread_join(watch_thread, NULL);
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&cond);
return 0;
}
```
###
阅读全文
相关推荐
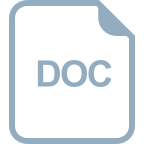
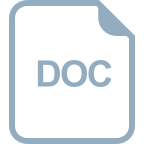

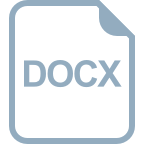
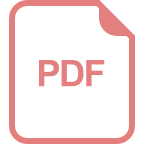
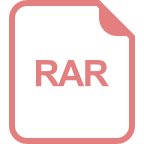
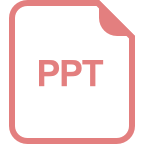
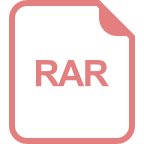
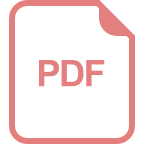
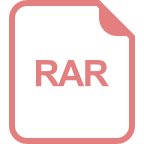
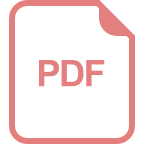
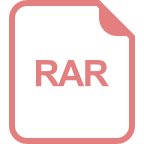
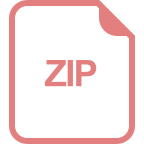
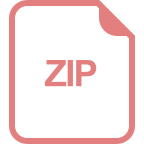
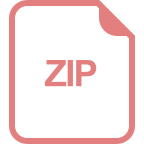
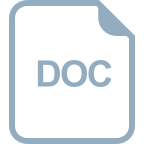
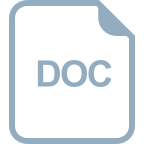
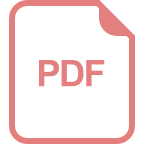