qt 检测流程里的 定时器怎么设置
时间: 2024-02-24 11:57:29 浏览: 12
在 Qt 的检测流程中,我们可以使用 QTimer 定时器来定时执行检测操作。定时器的设置步骤如下:
1. 在类的头文件中声明 QTimer 对象:`QTimer *m_timer;`
2. 在类的构造函数中创建 QTimer 对象,并设置定时器的时间间隔:`m_timer = new QTimer(this); m_timer->setInterval(1000);`
3. 连接 QTimer 对象的 timeout() 信号到槽函数:`connect(m_timer, SIGNAL(timeout()), this, SLOT(check()));`
4. 在槽函数中编写检测代码。
5. 调用 QTimer 对象的 start() 函数启动定时器:`m_timer->start();`
6. 如果需要停止定时器,调用 QTimer 对象的 stop() 函数:`m_timer->stop();`
下面是一个简单的示例代码,每隔一秒钟执行一次 check() 函数:
```cpp
#include <QApplication>
#include <QTimer>
#include <QDebug>
class MyDetector : public QObject
{
Q_OBJECT
public:
MyDetector(QObject *parent = nullptr) : QObject(parent)
{
m_timer = new QTimer(this);
m_timer->setInterval(1000);
connect(m_timer, SIGNAL(timeout()), this, SLOT(check()));
m_timer->start();
}
public slots:
void check()
{
qDebug() << "Checking...";
}
private:
QTimer *m_timer;
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
MyDetector detector;
return app.exec();
}
#include "main.moc"
```
在这个例子中,我们创建了一个名为 MyDetector 的类,这个类继承自 QObject。在 MyDetector 的构造函数中创建了一个 QTimer 对象,并设置其时间间隔为 1000 毫秒。然后通过 connect() 函数将 QTimer 对象的 timeout() 信号连接到 MyDetector 类的 check() 槽函数。在 check() 槽函数中,我们编写了检测代码。最后调用 start() 函数启动定时器,程序将会每秒钟执行一次 check() 函数,直到程序退出或者调用 stop() 函数停止定时器。
相关推荐
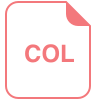
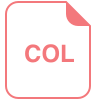
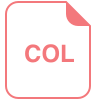
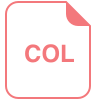
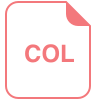









