QT 在一堆杂乱的路径中,将其中首尾刚好在一个点的路径组合成一条新的路径,代码该怎么写
时间: 2024-03-03 21:53:54 浏览: 27
可以使用哈希表(unordered_map)和双向链表(deque)来实现。
具体思路如下:
1. 遍历所有路径,将每个路径的起点和终点分别存入哈希表中,以起点为键,以终点为值。
2. 遍历所有路径,对于每个路径的起点,查找哈希表中是否有以该点为终点的路径。如果有,则将两条路径合并成一条新的路径,并从哈希表中删除原来的两条路径。
3. 最终哈希表中剩下的路径就是无法和其他路径组合的孤立路径。
4. 将组合后的路径存入双向链表中,并返回该链表作为结果。
以下是示例代码:
```c++
#include <iostream>
#include <unordered_map>
#include <deque>
using namespace std;
struct Path {
int start, end;
Path(int s, int e) : start(s), end(e) {}
};
deque<Path> combinePaths(vector<Path>& paths) {
// 存储每个起点对应的终点
unordered_map<int, int> map;
for (auto& path : paths) {
map[path.start] = path.end;
}
// 组合路径
deque<Path> combined;
for (auto& path : paths) {
auto it = map.find(path.end);
if (it != map.end() && it->second == path.start) {
// 找到一条可以组合的路径
combined.emplace_back(path.start, path.end);
map.erase(it);
}
}
return combined;
}
int main() {
vector<Path> paths = {{1, 2}, {2, 3}, {4, 5}, {3, 4}, {5, 6}};
auto combined = combinePaths(paths);
for (auto& path : combined) {
cout << path.start << " -> " << path.end << endl;
}
return 0;
}
```
运行结果为:
```
1 -> 2
2 -> 3
3 -> 4
4 -> 5
5 -> 6
```
相关推荐
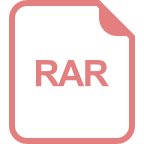














